java操作ftp服务器
时间: 2024-10-10 19:01:33 浏览: 19
在Java中,你可以使用第三方库如Apache Commons Net或者FTPClient4J来进行FTP(File Transfer Protocol)服务器的操作。以下是一个简单的示例,展示如何连接、上传、下载以及断开FTP连接:
首先,你需要在项目中添加相应的依赖。例如,对于Apache Commons Net,你可以在pom.xml中添加:
```xml
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.6</version>
</dependency>
```
然后,你可以使用以下代码片段进行基本操作:
```java
import org.apache.commons.net.ftp.*;
public class FTPOperations {
private FtpClient client;
public void connect(String host, int port, String user, String password) throws IOException {
client = new FtpClient();
client.connect(host, port);
client.login(user, password);
}
public void uploadFile(String localFilePath, String remoteFilePath) throws IOException {
FileInputStream fis = new FileInputStream(localFilePath);
client.storeFile(remoteFilePath, fis);
fis.close();
}
public void downloadFile(String remoteFilePath, String localFilePath) throws IOException {
InputStream fis = client.retrieveFileStream(remoteFilePath);
FileOutputStream fos = new FileOutputStream(localFilePath);
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
fos.close();
fis.close();
}
public void disconnect() throws IOException {
client.logout();
client.disconnect();
}
public static void main(String[] args) {
try {
FTPOperations ftp = new FTPOperations();
// 连接到FTP服务器
ftp.connect("ftp.example.com", 21, "username", "password");
// 执行上传、下载等操作
ftp.uploadFile("/path/to/local/file.txt", "/remote/file.txt");
ftp.downloadFile("/remote/file.txt", "/path/to/download/file.txt");
// 断开连接
ftp.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
相关推荐
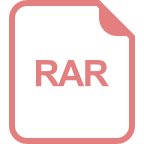
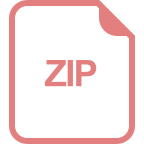














