C++首先定义一个子函数pf(int *x),用指针作为函数参数,在主函数中调用该函数,将变量值的地址传递给形参,算出该数的平方值,循环3次。
时间: 2024-10-14 08:08:58 浏览: 28
在C++中,你可以这样做:
```cpp
// 定义一个接受整型指针的子函数,用于计算传入指针指向数值的平方
void pf(int* x) {
// 计算指针所指向值的平方
int square = (*x) * (*x);
// 或者更简洁地,直接使用 std::pow(*x, 2)
// int square = std::pow(*x, 2);
// 输出或返回结果,这里假设函数不做实际输出,仅做处理
// ...
}
int main() {
// 创建一个整型变量并初始化它
int num = 5;
// 将变量的地址赋值给指针
int* ptr = #
// 循环三次,并在每次迭代中调用pf函数
for (int i = 0; i < 3; i++) {
// 调用pf函数,传递指针地址
pf(ptr);
// 如果需要,可以在这里更新指针或变量的值,然后继续下一次迭代
// 比如:ptr++; 或 num++;
}
return 0;
}
```
在这个例子中,`pf`函数接收了一个整型指针作为参数,通过解引用操作 `(*x)` 访问指针所指向的值,然后计算其平方。在`main`函数中,我们创建了一个`num`变量,并将其地址存储在`ptr`指针中。然后在循环里,我们将`ptr`传递给`pf`函数,实现了对`num`的平方值的操作。
相关问题
C++定义一个子函数pf(int *x),用指针作为函数参数,在主函数中调用该函数,将变量值的地址传递给形参,算出该数的平方值,循环3次。
在C++中,你可以通过定义一个接受指针作为参数的函数,并在主函数中传递变量的地址来完成这个任务。这里是一个简单的示例:
```cpp
// 定义子函数 pf,接收 int 类型指针并计算其指向数值的平方
void pf(int* x) {
if (x != nullptr) { // 验证指针是否为空
*x = (*x) * (*x); // 计算指针所指值的平方,并通过解引用操作赋值回去
} else {
std::cout << "Invalid pointer!" << std::endl;
}
}
int main() {
int numbers[] = {1, 2, 3}; // 创建一个整数数组
int size = sizeof(numbers) / sizeof(numbers[0]); // 获取数组长度
for (size_t i = 0; i < size; i++) {
pf(&numbers[i]); // 调用 pf 函数,传入数组元素的地址
std::cout << "Square of " << numbers[i] << " is: " << numbers[i] << std::endl;
}
return 0;
}
```
在这个例子中,`pf`函数接受一个`int`类型的指针,然后计算它所指的值(即数组元素)的平方,并更新指针所指向的值。在`main`函数里,我们遍历数组并将每个元素的地址传递给`pf`,以便计算并打印出它们的平方。
1. (简答题) 假设系统需要基于用户的输入执行相应的业务处理。请按下面的说明,完成一个C/C++程序的设计、编码和测试。 1。设业务处理函数有统一的声明形式:void process_name(int x, int y); 功能定义为两个整型数据的某种计算; 2。假定用户的输入为单个数字(从0到9),其中,9表示退出程序,其它的表示业务编号; 3。以用户输入为事件,则业务处理可视为相应的事件处理函数; 4。定义事件处理函数的函数指针数组; 5。基于用户输入为下标值,从函数指针数组中取出函数指针,并调用该它指向的函数。 提示: 1。定义一个函数指针变量:void (* pf)(int, int); //pf 是一个函数指针变量 2。定义一个函数指针类型:typedef void (* PtrFun)(int, int); //PrtFun 是一个函数指针类型。 PtrFun pf= nullptr; //定义一个函数指针变量pf,且它的值为空指针 PrtFun funs[9]; //定义一个函数指针数组,其中的每个元素指向一个类型的函数 3。更复杂的事件及其处理函数的关联机制可以使用map来表示和存储。 思考:上述过程也可以不使用函数指针,而是使用if语句来完成。试比较一个两者的区别。
下面是一个C++程序的设计、编码和测试,实现基于用户输入执行相应业务处理功能的过程:
```cpp
#include <iostream>
using namespace std;
// 业务处理函数的声明
void add(int x, int y);
void sub(int x, int y);
void mul(int x, int y);
void div(int x, int y);
// 函数指针类型的定义
typedef void (* PtrFun)(int, int);
// 函数指针变量和函数指针数组的定义
PtrFun pf = nullptr;
PtrFun funs[9] = {add, sub, mul, div};
// 业务处理函数的定义
void add(int x, int y)
{
cout << "x + y = " << x + y << endl;
}
void sub(int x, int y)
{
cout << "x - y = " << x - y << endl;
}
void mul(int x, int y)
{
cout << "x * y = " << x * y << endl;
}
void div(int x, int y)
{
if (y == 0)
{
cout << "Error: divide by zero!" << endl;
}
else
{
cout << "x / y = " << x / y << endl;
}
}
int main()
{
int choice;
while (true)
{
cout << "Please enter a number (0-9, 9 to quit): ";
cin >> choice;
if (choice == 9)
{
break;
}
else if (choice < 0 || choice > 3)
{
cout << "Error: invalid choice!" << endl;
}
else
{
int x, y;
cout << "Please enter two numbers: ";
cin >> x >> y;
pf = funs[choice - 1];
pf(x, y);
}
}
return 0;
}
```
上述程序中,定义了四个业务处理函数:`add`、`sub`、`mul`、`div`,它们的功能分别是两个整型数据的加、减、乘、除运算。定义了一个函数指针类型`PtrFun`,和一个函数指针变量`pf`和一个函数指针数组`funs`,其中函数指针数组的每个元素指向一个业务处理函数。通过用户输入的数字,确定调用哪个业务处理函数,从而实现相应的业务处理功能。
使用函数指针的优点是代码简洁,易于维护和扩展。使用if语句的实现方法也是可以的,但是随着业务处理函数的增多,if语句的嵌套会越来越深,代码难以维护和扩展。因此,使用函数指针是更好的选择。
阅读全文
相关推荐
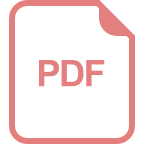
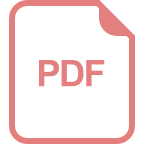
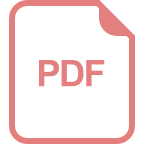

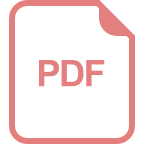
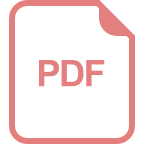
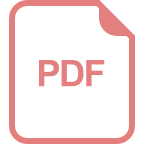
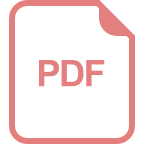
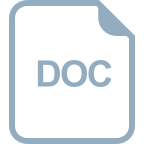
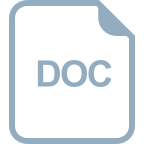
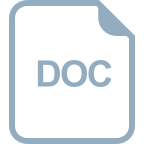
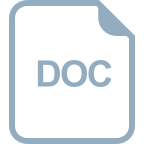
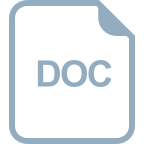
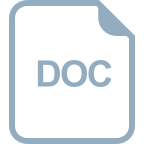

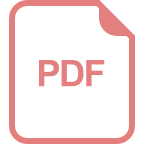