写一个多人聊天室的代码
时间: 2024-12-12 13:32:32 浏览: 4
编写一个简单的多人聊天室的代码示例通常涉及到网络编程,这里我会提供一个基于Python的简易版WebSocket服务器和客户端的框架,使用第三方库`websockets`。请注意这只是一个基础示例,实际应用可能需要更复杂的数据结构和错误处理。
首先,我们需要安装`websockets`库:
```bash
pip install websockets
```
### 服务器端(chat_server.py)
```python
import asyncio
from websockets import serve
async def handle_client(websocket, path):
message = await websocket.recv()
print(f"Received: {message}")
# 将消息广播给所有连接的客户端
for ws in clients:
if ws != websocket:
await ws.send(message)
clients = []
start_server = serve(handle_client, 'localhost', 8000)
asyncio.get_event_loop().run_until_complete(start_server)
print("Server is running on http://localhost:8000")
# 当有新的客户端连接时,将其添加到列表中
async def accept_connections(websocket, path):
clients.append(websocket)
try:
await handle_client(websocket, path)
finally:
clients.remove(websocket)
asyncio.get_event_loop().run_forever()
```
### 客户端(client.py)
```python
import websockets
import json
async def chat():
uri = "ws://localhost:8000"
async with websockets.connect(uri) as websocket:
name = input("Enter your name: ")
while True:
message = f"{name}: {input('Message: ')}"
await websocket.send(json.dumps({"type": "message", "data": message}))
response = await websocket.recv()
print(response)
if __name__ == "__main__":
asyncio.run(chat())
```
在这个例子中,服务器监听`localhost:8000`,当接收到新消息时,它会将消息广播给所有已连接的客户端。每个客户端都会显示其他人的消息。
**
阅读全文
相关推荐
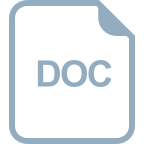
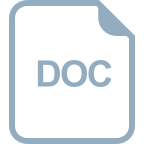
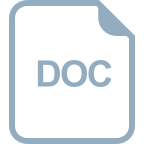

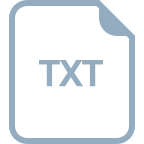
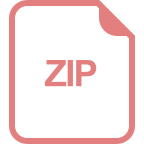










