qcustomplot跟随鼠标显示时间轴
时间: 2024-10-23 17:04:40 浏览: 36
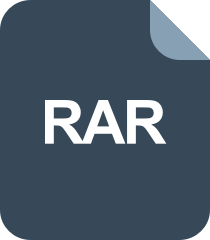
使用QCustomplot完成多Y轴曲线绘制

qCustomPlot是一个强大的Qt C++图表库,它允许用户创建定制化的绘图界面。如果你想要在qCustomPlot中实现跟随鼠标的时间轴显示,通常可以采用以下步骤:
1. 首先,在`QCPGraph`上设置X轴的行为,使其支持实时更新。你可以使用`QCPAxisTickerTime`来创建一个时间轴 ticker,并将其设置为当前时间。
```cpp
QCPAxis *xaxis = new QCPAxis(QCPAxis::Bottom);
xaxis->setTicker(new QCPAxisTickerTime());
```
2. 设置鼠标移动事件处理器,当鼠标悬停在图形上时,获取鼠标位置并计算对应的时间范围。
```cpp
QObject::connect(qcustomplot, &QCustomPlot::mouseMove, [this](const QPointF &pos) {
// 获取鼠标坐标对应的x值
QDateTime time = xaxis->tickedValue(xaxis->pixelToCoord(pos.x()));
// 更新时间轴文本显示或绘制新的区域
});
```
3. 可能需要在特定区域内绘制时间轴标签或其他信息,如使用`QCPRangePainter`。
4. 刷新图表以立即应用更改:
```cpp
qcustomplot->replot();
```
阅读全文
相关推荐
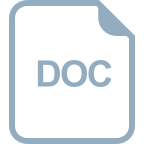
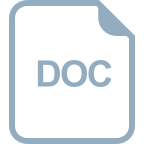
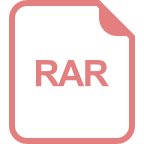
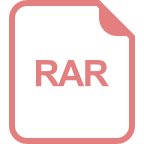
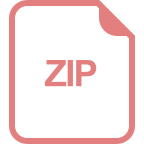
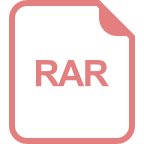










