make_shared C++
时间: 2023-08-05 21:05:11 浏览: 50
std::make_shared is a function template in C++ that is used to create a shared pointer to an object. It is defined in the <memory> header. Here's an example of how to use std::make_shared in C++:
```cpp
#include <iostream>
#include <memory>
class MyClass {
public:
MyClass() {
std::cout << "MyClass constructor called" << std::endl;
}
~MyClass() {
std::cout << "MyClass destructor called" << std::endl;
}
};
int main() {
std::shared_ptr<MyClass> ptr = std::make_shared<MyClass>();
// Access the object using the shared pointer
std::cout << "Using shared pointer" << std::endl;
return 0;
}
```
In the above example, std::make_shared is used to create a shared pointer to an instance of the MyClass class. The constructor of MyClass will be called when the shared pointer is created, and the destructor will be called when the shared pointer goes out of scope.
Note that std::make_shared is preferred over using the std::shared_ptr constructor directly because it can optimize memory allocation by combining memory allocation for the control block and the object into a single allocation.
相关推荐
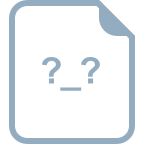
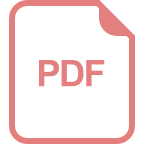
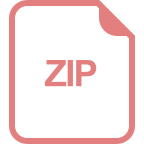














