vue-quill-editor图片拖拽
时间: 2023-08-31 22:13:25 浏览: 58
在Vue项目中使用vue-quill-editor插件实现图片拖拽,可以按照以下步骤进行操作:
1. 安装vue-quill-editor插件:
```
npm install vue-quill-editor --save
```
2. 在需要使用的组件中引入vue-quill-editor:
```javascript
import VueQuillEditor from 'vue-quill-editor'
import 'quill/dist/quill.core.css'
import 'quill/dist/quill.snow.css'
import 'quill/dist/quill.bubble.css'
```
3. 注册VueQuillEditor组件:
```javascript
export default {
components: {
VueQuillEditor
},
// ...
}
```
4. 在模板中使用VueQuillEditor组件:
```html
<template>
<div>
<vue-quill-editor v-model="content" :options="editorOptions"></vue-quill-editor>
</div>
</template>
```
5. 在data中定义content和editorOptions:
```javascript
data() {
return {
content: '',
editorOptions: {
// 其他配置项...
modules: {
toolbar: [
// 其他工具按钮...
[{ 'header': [1, 2, 3, 4, 5, 6, false] }],
['image']
],
// 添加拖拽上传配置
imageDrop: true,
imageResize: true
}
}
}
}
```
通过以上步骤,你就可以在vue-quill-editor中实现图片拖拽的功能了。记得根据自己的需求,配置合适的工具按钮和其他选项。
相关推荐
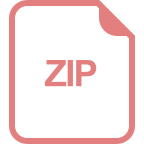
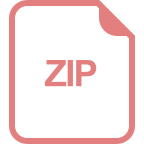














