can only concatenate str (not "xml.etree.ElementTree.Element") to str
时间: 2024-06-09 12:01:56 浏览: 299
这个错误提示是由于你在代码中尝试将一个字符串类型的变量与一个xml.etree.ElementTree.Element类型的变量进行拼接,而Python中只允许字符串与字符串进行拼接操作。
要解决这个问题,你可以将xml.etree.ElementTree.Element类型的变量转换为字符串类型,然后再进行拼接。可以使用ElementTree库中的tostring()方法将Element对象转换为字符串。
以下是一个示例代码:
```
import xml.etree.ElementTree as ET
# 创建一个Element对象
root = ET.Element("root")
child = ET.Element("child")
child.text = "Hello"
# 将Element对象转换为字符串
child_str = ET.tostring(child, encoding="unicode")
# 进行字符串拼接
result = "Prefix " + child_str + " Suffix"
print(result)
```
相关问题:
1. 什么是xml.etree.ElementTree.Element类型?
2. 如何将xml.etree.ElementTree.Element类型的变量转换为字符串?
3. Python中如何进行字符串拼接操作?
相关问题
can only concatenate str (not "lxml.etree._Element") to str
This error occurs when you are trying to concatenate a string with an object of type "lxml.etree._Element", which is not allowed. To concatenate the object with the string, you need to convert it to a string first. You can use the `str()` function to convert the object to a string. Here's an example:
```python
import lxml.etree as ET
# Assume element is an lxml.etree._Element object
element = ET.Element("tag")
# Convert the element to a string using ET.tostring() method
element_string = ET.tostring(element).decode()
# Concatenate the string with another string
result = "Some text " + element_string
print(result)
```
In this example, `ET.tostring()` method is used to convert the lxml.etree._Element object `element` to a string. The resulting string `element_string` can then be concatenated with another string using the `+` operator.
TypeError: can only concatenate str (not "lxml.etree._Element") to str
这个错误通常发生在你尝试将一个字符串和一个 `lxml.etree._Element` 对象进行连接操作时。Python中的字符串连接操作只能在两个字符串之间进行,而不能将其他类型的对象与字符串进行连接。
要解决这个错误,你需要将 `lxml.etree._Element` 对象转换为字符串。你可以使用 `lxml` 库中的 `tostring()` 方法将 `lxml.etree._Element` 对象转换为字符串。以下是一个示例:
```python
from lxml import etree
# 创建一个 lxml.etree._Element 对象
element = etree.Element("root")
element.text = "Hello, World!"
# 将 lxml.etree._Element 对象转换为字符串
element_str = etree.tostring(element).decode()
# 将字符串与另一个字符串连接
result = element_str + " more text"
print(result)
```
在上面的示例中,我们首先创建了一个 `lxml.etree._Element` 对象 `element`,然后使用 `etree.tostring()` 方法将其转换为字符串 `element_str`。最后,我们将 `element_str` 与另一个字符串进行连接,并打印结果。
请注意,`etree.tostring()` 方法返回的是字节字符串,因此我们使用 `decode()` 方法将其解码为普通字符串。
希望这能帮助到你!如果你有任何其他问题,请随时问。
阅读全文
相关推荐
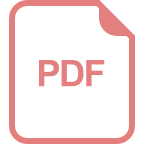
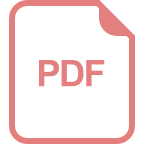













