在idea中编写一个java代码实现将数据库中goods表格内的内容打印出来
时间: 2024-12-04 12:23:05 浏览: 12
在IntelliJ IDEA中编写Java代码来打印数据库中goods表的内容,通常需要使用JDBC(Java Database Connectivity)连接数据库并执行SQL查询。以下是简单的步骤和代码示例:
首先,确保已经添加了数据库驱动依赖(如MySQL的jtds、ojdbc等),并在`pom.xml`中配置好。
```xml
<dependencies>
<dependency>
<groupId>com.mysql.jdbc</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version> <!-- 更新到你的实际版本 -->
</dependency>
</dependencies>
```
然后,创建一个Java类,例如`DatabasePrinter`,并设置数据库连接信息:
```java
import java.sql.*;
public class DatabasePrinter {
private String url = "jdbc:mysql://localhost:3306/your_database"; // 数据库地址
private String username = "root";
private String password = "your_password";
public void printGoods() {
try {
Connection connection = DriverManager.getConnection(url, username, password);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM goods");
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
double price = resultSet.getDouble("price");
System.out.println("ID: " + id + ", Name: " + name + ", Price: " + price);
}
resultSet.close();
statement.close();
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
最后,在主函数中实例化这个类并调用方法:
```java
public static void main(String[] args) {
DatabasePrinter printer = new DatabasePrinter();
printer.printGoods();
}
```
这将打印出`goods`表中的所有行。别忘了替换上述代码中的数据库URL、用户名和密码为实际值。
阅读全文
相关推荐




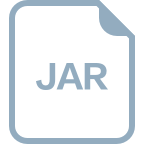













