使用C++实现编写一个函数,接受一个字符串作为参数,计算该字符串中每个字符出现的次数,并将结果存储在一个数组中返回
时间: 2024-10-22 14:19:33 浏览: 30
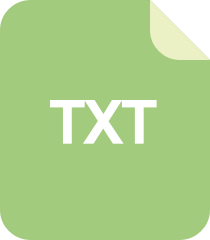
C/C++面试之算法系列--几个典型的内存拷贝及字符串函数实现

在C++中,你可以使用`std::map`或者自定义数组和计数器来实现这个功能。这里给出一个使用`std::map`的例子,它会统计每个字符的出现次数,并存储在`std::map<char, int>`类型的结构中:
```cpp
#include <string>
#include <map>
// 函数声明
std::map<char, int> countCharacters(const std::string& input);
// 实现函数
std::map<char, int> countCharacters(const std::string& input) {
std::map<char, int> charCounts;
for (char c : input) {
// 如果字符已经在map中,计数加一;否则,初始化计数为1
if (charCounts.find(c) != charCounts.end()) {
charCounts[c]++;
} else {
charCounts[c] = 1;
}
}
return charCounts;
}
```
如果你想返回一个固定大小的数组,可以使用`std::vector<int>`,并动态调整它的大小。注意,这种方法需要额外的步骤来管理数组大小和填充值:
```cpp
#include <string>
#include <vector>
// 函数声明
std::vector<int> countCharactersInArray(const std::string& input, size_t maxLength = 256);
// 实现函数
std::vector<int> countCharactersInArray(const std::string& input, size_t maxLength = 256) {
std::vector<int> counts(maxLength, 0);
for (char c : input) {
if (c >= 'a' && c <= 'z' || c >= 'A' && c <= 'Z') { // 只统计ASCII字母,假设你想忽略其他字符
counts[c - 'a']++; // 对小写字母进行索引,以便使用0到25的范围
}
}
return counts;
}
```
在这个例子中,我们假设输入仅包含ASCII字母,如果是全英文字符集或其他情况,可能需要调整条件。
阅读全文
相关推荐
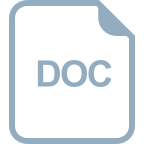
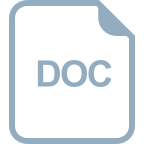
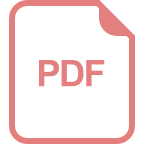
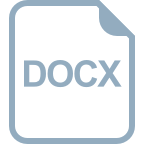
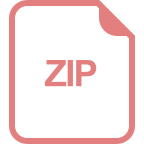
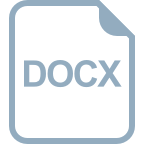
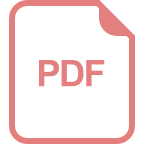
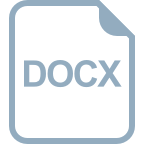
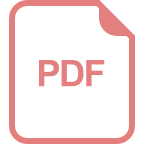
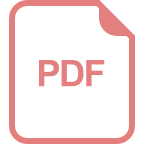
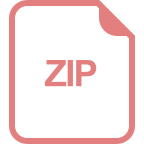
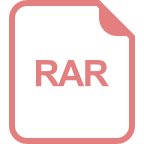
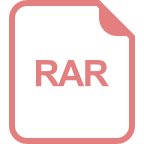
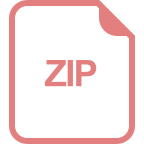
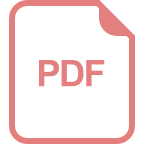
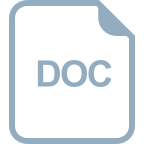