vxe-select 限制多选个数
时间: 2024-09-18 21:14:32 浏览: 62
vxe-select是Vue Element Plus库中的下拉选择组件,它允许用户进行多选操作,并提供了一定程度的自定义选项。如果你想在vxe-select中限制用户可以选择的最大项数,你可以通过设置`max`属性来实现这个功能。
例如,在HTML模板中,你可以这样做:
```html
<vxe-select
max="5" <!-- 这里设置了最多只能选择5项 -->
:options="items" <!-- items是你数据源里的选项数组 -->
></vxe-select>
```
在这个例子中,如果用户尝试选择超过5个选项,超出部分将不会显示在列表中,并且组件会阻止进一步的选择。
如果你想要动态控制最大值,也可以在JavaScript中设置:
```js
data() {
return {
maxChoices: 5, // 初始化最大选择数
};
},
methods: {
handleMaxChange(value) {
this.maxChoices = value; // 当需要改变最大值时,更新这个属性
},
}
```
然后在模板上绑定`max`到`handleMaxChange`方法:
```html
<vxe-select
@max-changed="handleMaxChange"
max="maxChoices"
:options="items"
></vxe-select>
```
相关问题
vxe-table实现多选
要在vxe-table中实现多选,可以在列定义中使用`type: 'checkbox'`来定义一个多选列,然后在表格的头部和每一行中显示多选框。在表格中选中的行将会被添加到一个名为`$refs.table.getCheckboxRecords()`的数组中。
以下是一个示例代码:
```
<template>
<div>
<vxe-table ref="table" :data="tableData" :columns="columns">
<template #toolbar>
<div>
<button @click="handleDelete">删除选中行</button>
</div>
</template>
<template #checkbox="params">
<input type="checkbox" :checked="params.row._checked" @change="handleCheckboxChange(params.row)">
</template>
</vxe-table>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [
{ id: 1, name: '张三' },
{ id: 2, name: '李四' },
{ id: 3, name: '王五' },
],
columns: [
{ type: 'checkbox', width: 60, align: 'center' },
{ field: 'id', title: 'ID', width: 80 },
{ field: 'name', title: '姓名' },
],
selectedRows: []
}
},
methods: {
handleCheckboxChange(row) {
row._checked = !row._checked
this.selectedRows = this.$refs.table.getCheckboxRecords()
},
handleDelete() {
const ids = this.selectedRows.map(row => row.id)
this.tableData = this.tableData.filter(row => !ids.includes(row.id))
this.selectedRows = []
}
}
}
</script>
```
在上面的代码中,我们定义了一个名为`selectedRows`的数组,用于存储选中的行。在表格的列定义中,我们使用`type: 'checkbox'`来定义一个多选列,并使用`template #checkbox`来定义多选框的显示样式。在多选框的`@change`事件中,我们将选中状态存储到行的`_checked`属性中,并调用`this.$refs.table.getCheckboxRecords()`获取选中的行。在删除选中行的按钮的点击事件中,我们先获取选中行的ID,然后使用`filter`方法从数据中删除被选中的行。最后,我们清空`selectedRows`数组。
用 vxe-table 实现 多选下拉表格
1. 安装 vxe-table
```
npm install vxe-table --save
```
2. 在 Vue 项目中引用 vxe-table
```javascript
import Vue from 'vue'
import 'xe-utils'
import VXETable from 'vxe-table'
import 'vxe-table/lib/style.css'
Vue.use(VXETable)
```
3. 在 Vue 模板中使用 vxe-table
```html
<vxe-table :data="tableData" checkbox-config="{range: true}">
<vxe-table-column type="selection" width="60"></vxe-table-column>
<vxe-table-column field="name" title="姓名"></vxe-table-column>
<vxe-table-column field="age" title="年龄"></vxe-table-column>
<vxe-table-column field="address" title="地址"></vxe-table-column>
</vxe-table>
```
4. 在 Vue 实例中定义数据
```javascript
export default {
data() {
return {
tableData: [
{ name: '张三', age: 20, address: '北京市' },
{ name: '李四', age: 30, address: '上海市' },
{ name: '王五', age: 40, address: '广州市' },
{ name: '赵六', age: 50, address: '深圳市' }
]
}
}
}
```
5. 使用多选下拉框
```html
<template>
<div>
<vxe-dropdown ref="dropdown" v-model="value" :options="options" multiple>
<template #option="{ option, $index }">
<vxe-checkbox :label="option.value" :content="option.label" :indeterminate="option.indeterminate" :checked="option.checked" @change="handleChange($event, option)"></vxe-checkbox>
</template>
</vxe-dropdown>
<vxe-table :data="tableData" checkbox-config="{range: true}" ref="table">
<vxe-table-column type="selection" width="60"></vxe-table-column>
<vxe-table-column field="name" title="姓名"></vxe-table-column>
<vxe-table-column field="age" title="年龄"></vxe-table-column>
<vxe-table-column field="address" title="地址"></vxe-table-column>
</vxe-table>
</div>
</template>
```
```javascript
export default {
data() {
return {
options: [
{ label: '张三', value: '1' },
{ label: '李四', value: '2' },
{ label: '王五', value: '3' },
{ label: '赵六', value: '4' },
],
value: ['1', '2'],
tableData: [
{ name: '张三', age: 20, address: '北京市' },
{ name: '李四', age: 30, address: '上海市' },
{ name: '王五', age: 40, address: '广州市' },
{ name: '赵六', age: 50, address: '深圳市' }
]
}
},
methods: {
handleChange (checked, option) {
if (checked) {
this.value.push(option.value)
} else {
const index = this.value.indexOf(option.value)
if (index > -1) {
this.value.splice(index, 1)
}
}
}
}
}
```
阅读全文
相关推荐
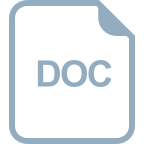
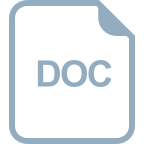
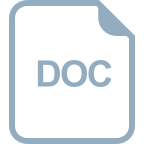













