C语言实现带头结点的单链表的最小元素插入最前面 且不申请新的结点
时间: 2024-10-21 21:01:57 浏览: 42
在C语言中,如果我们想要在单链表头部插入一个新结点以保持链表中最小元素的位置,我们可以采用一种称为“头插法”的策略,并在查找最小值的同时插入。以下是简单的步骤:
1. 定义链表结构体,包含数据域`data`和指向下一个节点的指针`next`。
```c
typedef struct ListNode {
int data;
struct ListNode *next;
} ListNode;
```
2. 创建一个辅助函数,用于找到当前链表中的最小值。假设链表非空。
```c
ListNode* findMin(ListNode* head) {
if (head == NULL || head->next == NULL)
return head; // 如果链表为空,返回头节点
ListNode* minNode = head;
while (minNode->next != NULL) {
if (minNode->data > minNode->next->data)
minNode = minNode->next;
}
return minNode;
}
```
3. 插入操作:当有新元素需要插入时,首先找到最小值,然后将新元素插入到该位置之前。
```c
void insertAtMin(int value, ListNode** headRef) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode)); // 新建节点
newNode->data = value;
ListNode* minNode = findMin(*headRef); // 查找最小值节点
newNode->next = *headRef; // 将新节点链接到头节点前
if (minNode == *headRef) { // 如果最小值就是头节点
*headRef = newNode;
} else {
ListNode* current = *headRef;
while (current->next != minNode) {
current = current->next;
}
current->next = newNode;
}
}
```
4. 使用这个`insertAtMin`函数插入新值,例如:
```c
int main() {
ListNode* head = NULL; // 初始化链表为空
// ...其他链表操作...
insertAtMin(value, &head);
// ...继续链表操作...
return 0;
}
```
阅读全文
相关推荐
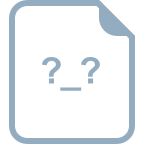
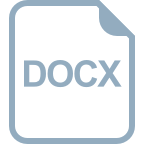
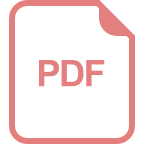




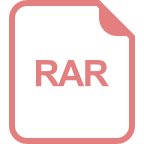
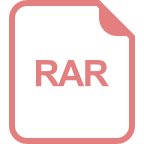
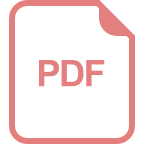
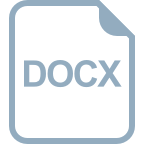
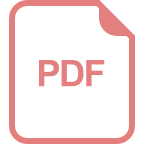
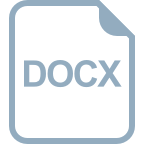
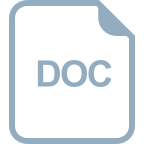
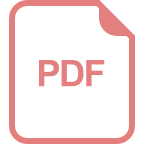
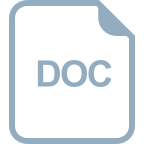
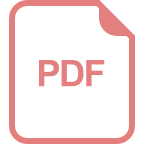

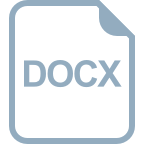
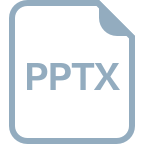