gillespie算法
时间: 2023-12-01 22:43:19 浏览: 12
Gillespie算法是一种用于模拟化学反应动力学的随机模拟算法。它基于化学反应速率常数和反应物浓度,通过随机选择反应类型和时间来模拟化学反应的动力学过程。Gillespie算法的核心思想是将化学反应看作是一系列随机事件的集合,每个事件都有一个特定的速率常数和反应物浓度。在每个时间步长内,Gillespie算法随机选择一个事件,并计算该事件发生的概率。如果该事件发生,则更新反应物浓度并继续模拟下一个时间步长。如果该事件未发生,则继续选择下一个事件并计算其发生概率,直到有一个事件发生为止。
以下是一个使用Python实现Gillespie算法的简单示例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义反应速率常数和反应物浓度
k1 = 0.1
k2 = 0.01
A0 = 100
B0 = 200
# 定义反应类型和速率常数
reactions = [
{'reactants': {'A': 1, 'B': 1}, 'products': {'C': 1}, 'rate': k1},
{'reactants': {'C': 1}, 'products': {'A': 1, 'B': 1}, 'rate': k2}
]
# 定义模拟参数
t_max = 100
n_steps = 1000
# 初始化反应物浓度和时间
t = np.linspace(0, t_max, n_steps)
A = np.zeros_like(t)
B = np.zeros_like(t)
C = np.zeros_like(t)
A[0] = A0
B[0] = B0
# 运行Gillespie算法模拟化学反应
for i in range(1, n_steps):
# 计算每个反应的速率
rates = [reaction['rate'] * A[i-1]**reaction['reactants'].get('A', 0) * B[i-1]**reaction['reactants'].get('B', 0) * C[i-1]**reaction['reactants'].get('C', 0) for reaction in reactions]
# 计算总速率
total_rate = sum(rates)
# 随机选择一个反应
reaction_index = np.random.choice(len(reactions), p=[rate/total_rate for rate in rates])
# 更新反应物浓度
for reactant, stoichiometry in reactions[reaction_index]['reactants'].items():
exec(f"{reactant}[i] = {reactant}[i-1] - {stoichiometry}")
for product, stoichiometry in reactions[reaction_index]['products'].items():
exec(f"{product}[i] = {product}[i-1] + {stoichiometry}")
# 绘制反应物浓度随时间的变化曲线
plt.plot(t, A, label='A')
plt.plot(t, B, label='B')
plt.plot(t, C, label='C')
plt.xlabel('Time')
plt.ylabel('Concentration')
plt.legend()
plt.show()
```
相关推荐
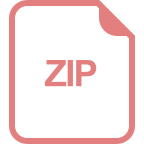
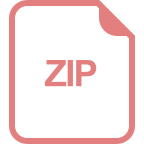



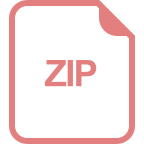
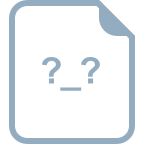
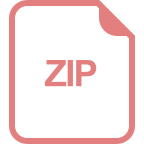
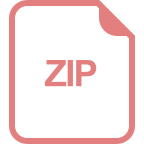
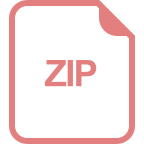
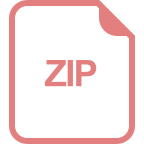
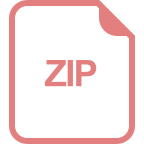
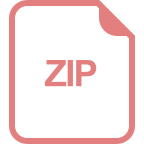