如何在百度地图(vue-baidu-map)中实现,当鼠标悬停时仅显示特定的BmPointCollection中的一个点并保持其隐藏状态直到鼠标移入?
时间: 2024-12-24 10:39:46 浏览: 6
在Vue.js结合百度地图API(vue-baidu-map)中实现这个功能,你需要在组件内部处理地图事件和BmPointCollection的管理。以下是一个简单的步骤说明:
1. 首先,在`data()`函数中初始化你需要的变量,例如一个数组来存储选中的点,以及一个标志来追踪当前是否有一个点处于高亮状态。
```javascript
data() {
return {
selectedPoint: null,
highlightEnabled: false,
points: [] // 假设这是你的BmPointCollection实例
}
}
```
2. 当你创建或初始化BmPointCollection时,确保它有唯一的标识符或可以设置一个标识符。
3. 定义一个方法来处理鼠标悬停事件,比如`handleMouseHover(e, point)`。在这个方法里,检查鼠标是否悬停在一个点上,并更新`selectedPoint`和`highlightEnabled`的状态。
```javascript
methods: {
handleMouseHover(e, point) {
if (this.highlightEnabled && this.selectedPoint !== point) {
this.points[this.selectedPoint].setHighlight(false);
}
if (!this.highlightEnabled || this.selectedPoint !== point) {
this.selectedPoint = point;
this.points[point.id].setHighlight(true); // 假设每个点都有一个id属性
this.highlightEnabled = true;
} else {
// 如果已经选择了一个点并且再次悬停,取消高亮
this.points[point.id].setHighlight(false);
this.highlightEnabled = false;
}
}
}
```
4. 在地图元素上监听鼠标移动事件,并将`handleMouseHover`作为回调传递给该事件:
```html
<template>
<baidu-map :center="mapCenter" @dragend="handleMapDragEnd">
<bm-point-collection v-for="(point, index) in points" :key="index"
:position="point.position"
:hover-event="handleMouseHover"
:point-id="index" /> <!-- 这里的`hover-event`和`point-id`假设你是通过索引来标识点 -->
</baidu-map>
</template>
<script>
...
export default {
...
methods: {
...
},
mounted() {
this.handleMapDragEnd();
}
...
}
</script>
```
5. 最后,记得清理已添加的鼠标移动监听器,以防内存泄漏。在`beforeDestroy`生命周期钩子中执行此操作:
```javascript
beforeDestroy() {
this.$el.removeEventListener('mousemove', this.handleMouseHover);
}
```
现在,当你在地图上移动鼠标时,只有鼠标悬停的那个点会被高亮显示,其他点会保持隐藏状态直到鼠标离开。如果需要在鼠标移出时清除高亮,可以在`handleMouseHover`方法中加入相应的逻辑。
阅读全文
相关推荐
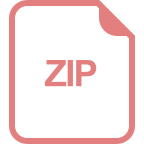
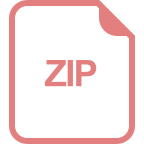
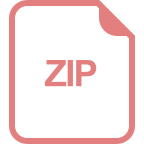



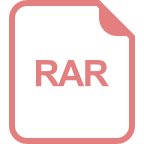
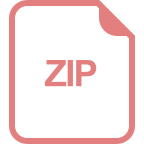
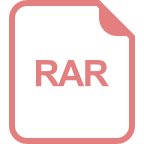
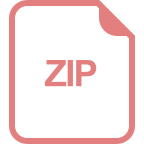
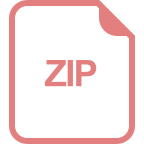
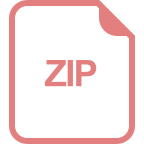
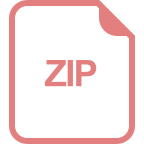
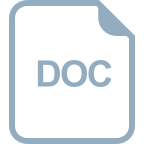
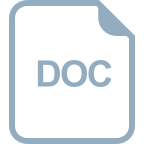
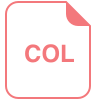
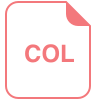

