用c语言编程从键盘上输入5个字符串(约定:每个字符串中字符数≤80字节),对其进行升序排序并输出。A[5][80]
时间: 2024-10-11 10:02:55 浏览: 24
在C语言中,我们可以使用数组来存储这五个字符串,并利用`strcmp()`函数来进行字符串比较,然后结合冒泡排序算法对它们进行升序排列。以下是简单的步骤:
1. 首先,我们需要创建一个二维字符数组 `A[5][80]` 来存储输入的字符串。
2. 使用`for`循环从键盘获取用户输入的5个字符串,并将其复制到数组中。
3. 冒泡排序函数遍历数组,对于每一对相邻的元素,如果顺序错误就交换它们的位置,直到整个数组都有序。
4. 最后,打印排序后的字符串。
下面是实现这一功能的示例代码:
```c
#include <stdio.h>
#include <string.h>
void bubbleSort(char A[][80], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (strcmp(A[j], A[j + 1]) > 0) {
// 交换字符串
char temp[80];
strcpy(temp, A[j]);
strcpy(A[j], A[j + 1]);
strcpy(A[j + 1], temp);
}
}
}
}
int main() {
char A[5][80];
int count = 0;
while (count < 5) {
printf("请输入第 %d 个字符串(不超过80字节): ", count + 1);
fgets(A[count], sizeof(A[0]), stdin); // 读取一行字符串,去掉换行符
A[count][strcspn(A[count], "\n")] = '\0'; // 去掉换行符
count++;
}
bubbleSort(A, 5);
printf("升序排列后的字符串:\n");
for (int i = 0; i < 5; i++) {
printf("%s\n", A[i]);
}
return 0;
}
```
阅读全文
相关推荐
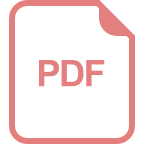
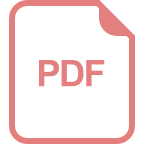
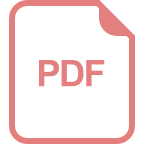















