python 查找文本中关键字出现次数
时间: 2023-11-27 21:01:11 浏览: 96
要使用Python查找文本中关键字出现的次数,可以使用字符串的`count`方法来实现。下面是一个例子:
```python
def count_keywords(text, keyword):
count = text.count(keyword)
return count
# 示例用法
text = "Python是一种简单易学但功能强大的编程语言,用于数据分析,人工智能和Web开发。"
keyword = "Python"
result = count_keywords(text, keyword)
print(f"关键字 \"{keyword}\" 出现了 {result} 次。")
```
上面的代码定义了一个函数`count_keywords`,接受两个参数:`text`是要搜索的文本,`keyword`是要查找的关键字。使用`text.count(keyword)`可以统计关键字在文本中出现的次数,该函数返回结果。
在示例中,我们定义了一个文本字符串`text`,包含了一个关键字`keyword`,然后调用`count_keywords`函数,将文本和关键字作为参数传入。最后打印结果就可以得知关键字在文本中出现的次数。
相关问题
selenium查找页面表格某一列中关键字出现次数
可以通过以下步骤实现:
1. 使用 Selenium 打开页面并找到表格元素。
2. 遍历表格行,获取每行目标列的文本内容。
3. 利用 Python 的字符串方法 count() 统计目标关键字在文本中出现的次数。
4. 累加每行中目标关键字出现的次数,最后输出总数。
示例代码:
```python
from selenium import webdriver
# 打开页面
driver = webdriver.Chrome()
driver.get('https://example.com')
# 找到表格元素
table = driver.find_element_by_css_selector('table')
# 目标列索引(从0开始)
target_col_index = 1
# 目标关键字
target_keyword = 'apple'
# 初始化出现次数
count = 0
# 遍历表格行
for row in table.find_elements_by_css_selector('tr'):
# 获取目标列文本
target_text = row.find_elements_by_css_selector('td')[target_col_index].text
# 统计出现次数
count += target_text.count(target_keyword)
# 输出总次数
print(f'Total count: {count}')
```
注意,此方法只适用于静态页面。如果表格内容是通过 JavaScript 动态加载的,需要使用 Selenium 的等待机制确保表格内容已经加载完成。
python如何快速查找关键字
在 Python 中可以使用 `in` 关键字来查找一个字符串中是否包含一个关键字,例如:
```python
text = "这是一段文本,其中包含了关键字Python"
if "Python" in text:
print("找到关键字Python")
else:
print("未找到关键字Python")
```
此外,如果需要在一个列表或者字典中查找关键字,可以使用 `in` 关键字,例如:
```python
# 在列表中查找关键字
keywords = ["Python", "Java", "C++"]
if "Python" in keywords:
print("找到关键字Python")
else:
print("未找到关键字Python")
# 在字典中查找关键字
keyword_dict = {"name": "Python", "age": 30, "location": "Beijing"}
if "Python" in keyword_dict.values():
print("找到关键字Python")
else:
print("未找到关键字Python")
```
使用以上方法可以快速查找关键字。
相关推荐
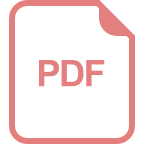
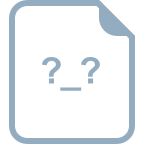
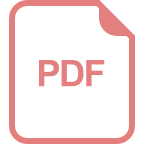
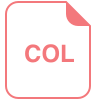
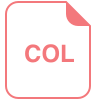










