选择排序JAVA代码
时间: 2024-11-06 09:11:27 浏览: 13
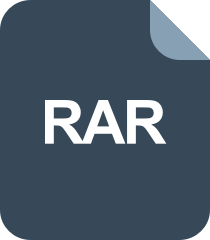
选择排序java 代码

选择排序(Selection Sort)是一种简单直观的排序算法,它的工作原理是每一次从未排序的数据元素中找到最小(或最大)的一个,存放到已排序序列的末尾。这是一种比较型排序算法,时间复杂度为O(n^2)。
以下是使用Java编写的选择排序示例:
```java
public class SelectionSort {
void selectionSort(int arr[]) {
int n = arr.length;
// One by one move boundary of unsorted subarray
for (int i = 0; i < n - 1; i++) {
// Find the minimum element in unsorted array
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j; // update index of minimum element
}
}
// Swap the found minimum element with the first element of the unsorted part
int temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
}
}
// Function to print an array
void printArray(int arr[]) {
int n = arr.length;
for (int i=0; i<n; ++i)
System.out.print(arr[i] + " ");
System.out.println();
}
public static void main(String args[]) {
SelectionSort ob = new SelectionSort();
int arr[] = {64, 25, 12, 22, 11};
ob.selectionSort(arr);
System.out.println("Sorted array");
ob.printArray(arr);
}
}
```
在这个例子中,`selectionSort()` 方法实现了选择排序,首先初始化一个未排序数组 `arr`,然后通过两个嵌套循环找到最小值并交换位置。`printArray()` 方法用于打印排序后的数组。在 `main()` 函数中,我们创建了一个 `SelectionSort` 对象并测试了这个排序算法。
阅读全文
相关推荐
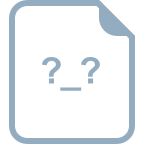
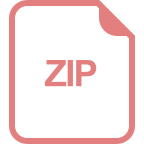
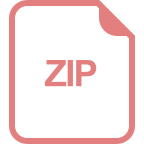
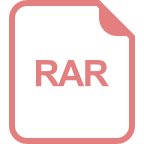
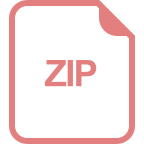
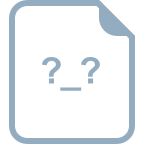
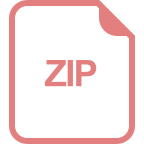
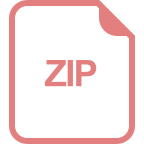
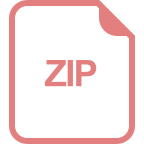
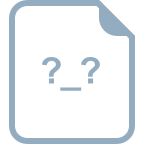
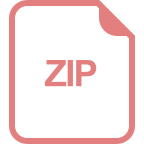
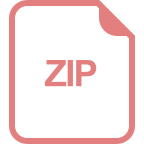
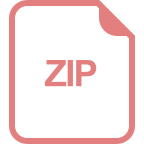
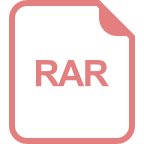
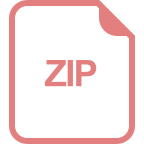
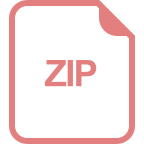