基于python求数字理想低通滤波器和理想高通滤波器的IDTFT,绘制时域序列图
时间: 2024-12-14 12:24:34 浏览: 6
在Python中,可以使用NumPy库来进行数字信号处理,包括理想的低通(LPF)和高通(HPF)滤波器的设计,然后利用快速傅里叶变换(IFFT)计算其逆傅立叶变换(IDTFT),得到时域序列。下面是一个简化的步骤:
1. 导入必要的库:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.signal import firwin, freqz
```
2. 设定采样率、滤波器截止频率和滤波器长度:
```python
fs = 44100 # 采样频率,例如CD质量音频
cutoff_freq = 500 # 截止频率 (Hz)
filter_order = 8 # 滤波器阶数
```
3. 计算理想滤波器的firwin函数:
```python
def ideal_filter(filter_type, cutoff, fs, order):
if filter_type == 'lowpass':
b = firwin(order, cutoff / (fs / 2), pass_zero=True, pass_band=[0, cutoff], stop_band=[cutoff, fs / 2])
elif filter_type == 'highpass':
b = firwin(order, cutoff / (fs / 2), pass_zero=False, stop_band=[0, cutoff])
else:
raise ValueError("Invalid filter type")
return b
# 获取低通或高通滤波器系数
b_low = ideal_filter('lowpass', cutoff_freq, fs, filter_order)
b_high = ideal_filter('highpass', cutoff_freq, fs, filter_order)
```
4. 使用IDTFT并绘制频谱和时域图:
```python
n_taps = len(b_low) # 滤波器长度
t = np.arange(-n_taps // 2, n_taps // 2 + 1) / fs # 时间轴
# IDTFT
x_low = np.fft.ifft(b_low)
x_high = np.fft.ifft(b_high)
# 绘制频谱图
freqs, responses = freqz(b_low, worN=8000)
plt.figure()
plt.plot(freqs, 20 * np.log10(np.abs(responses)), label='Lowpass')
freqs, responses = freqz(b_high, worN=8000)
plt.plot(freqs, 20 * np.log10(np.abs(responses)), label='Highpass')
plt.xlabel('Frequency (Hz)')
plt.ylabel('Magnitude (dB)')
plt.title(f'Ideal Filter Frequency Response - LPF and HPF @ {cutoff_freq} Hz')
plt.legend()
# 绘制时域序列图
plt.figure()
plt.subplot(2, 1, 1)
plt.plot(t, np.real(x_low), label=f'Lowpass Output')
plt.subplot(2, 1, 2)
plt.plot(t, np.real(x_high), label=f'Highpass Output')
plt.xlabel('Time (s)')
plt.show()
```
这个例子展示了如何基于Python设计理想低通和高通滤波器,并通过IDTFT将其转换到时域展示出来。运行此代码将生成两个图表:一个显示了滤波器的频率响应,另一个展示了滤波后的时域信号。
阅读全文
相关推荐
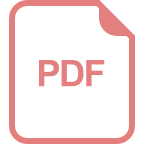
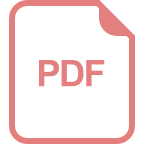
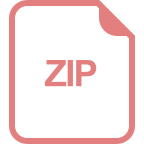
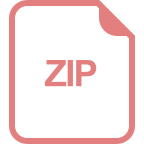
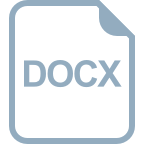
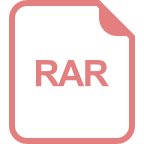