C++ unordered_map count方法
时间: 2024-09-23 07:01:28 浏览: 41
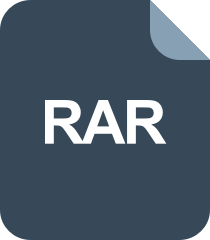
unordered-map的使用方法.rar
在C++标准库中,`unordered_map`是一个关联容器,它提供了键值对的无序存储。当你需要快速查找元素是否存在以及其出现次数时,可以使用它的`count`方法。
`count(key)`函数用于返回指定键对应的元素数量。如果键存在,则返回1;如果键不存在于映射中,则返回0。这个操作的时间复杂度通常是O(1),因为哈希表的查找非常高效。
例如:
```cpp
std::unordered_map<int, std::string> myMap;
// 插入一些元素
myMap[1] = "one";
myMap[2] = "two";
int countOfOne = myMap.count(1); // countOfOne将被设置为1
if (countOfOne > 0) {
std::cout << "Key 1 exists and appears " << countOfOne << " times." << std::endl;
} else {
std::cout << "Key 1 does not exist." << std::endl;
}
```
阅读全文
相关推荐
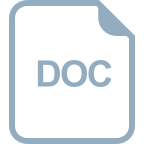
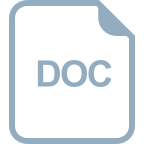















