strcpy 使用数组
时间: 2023-12-20 10:29:41 浏览: 59
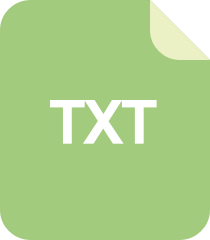
strcpy函数
可以使用数组和strcpy函数来进行字符串的赋值操作。下面是一个例子:
```c
#include <stdio.h>
#include <string.h>
int main () {
char str1[20];
char str2[20];
char str3[20];
int len ;
strcpy(str1, "hello");
strcpy(str2, "world");
strcpy(str3, str1);
printf("str1: %s\n", str1);
printf("str2: %s\n", str2);
printf("str3: %s\n", str3);
len = strlen(str1);
printf("Length of str1: %d\n", len);
return 0;
}
```
输出结果为:
```
str1: hello
str2: world
str3: hello
Length of str1: 5
```
在上面的例子中,我们定义了三个字符数组str1、str2和str3,然后使用strcpy函数将字符串"hello"和"world"分别赋值给str1和str2,将str1的值复制给str3。最后,我们使用strlen函数计算了str1的长度并将其输出。
阅读全文
相关推荐
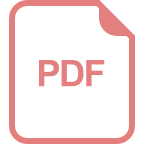















