编写一个程序,至少创建2个线程,两个线程都循环执行,一个线程每隔1秒输出我是线程1,另一个线程每隔1秒输出我是线程2 Linux环境下 用POSIX线程库创建并管理线程
时间: 2024-09-26 11:01:24 浏览: 29
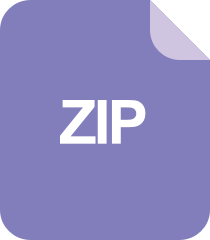
易语言多线程多次启动一个子程序
在Linux环境下,我们可以利用POSIX线程库(也称为Pthreads)编写一个简单的多线程程序,让两个线程交替输出。以下是一个基本的C语言示例:
```c
#include <stdio.h>
#include <pthread.h>
#include <time.h>
// 定义全局计数器和线程ID
static int counter = 0;
static pthread_t thread1, thread2;
void *thread_function(void *arg) {
int tid = (int)arg;
while (counter < 5) { // 为了演示,我们限制每个线程只运行5次
printf("我是线程%ld,当前时间戳:%lu\n", tid, time(NULL));
if (tid == 1)
sleep(1); // 线程1每秒打印一次
else
sleep(2); // 线程2每两秒打印一次,以便交替
++counter;
}
pthread_exit(NULL);
}
int main() {
pthread_create(&thread1, NULL, thread_function, (void *)1);
pthread_create(&thread2, NULL, thread_function, (void *)2);
// 主线程等待两个子线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
return 0;
}
```
在这个例子中,`thread_function`是一个线程函数,它接收一个整数作为参数,代表线程编号。主函数首先创建两个线程,然后通过`pthread_join`等待它们各自完成。
当你运行这个程序,你应该能看到"我是线程1"和"我是线程2"交替显示,每次间隔大约1秒(对于线程1),而线程2隔2秒才打印一次。
阅读全文
相关推荐
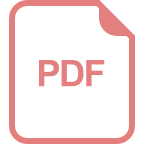
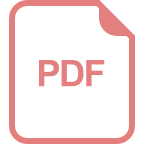



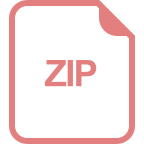
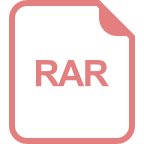
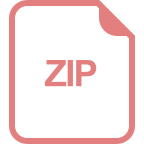
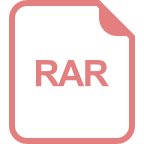
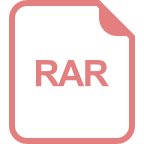
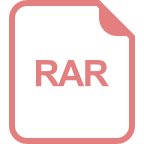
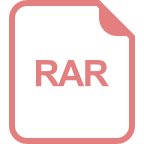
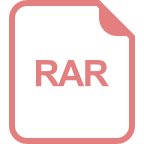
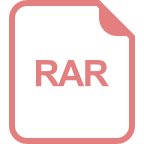
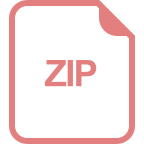
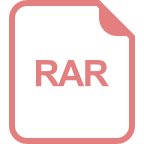
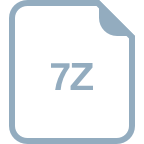