请用【java】帮我写一段快排代码,并帮我讲解下实现逻辑长方形和圆形都属于几何图形,都有周长和面积,并且它们都有自己的周长和面积计算公式。使用多态的知识设计程序可以计算不同图形的面积和周长
时间: 2024-11-03 08:23:26 浏览: 40
在Java中,我们可以使用多态(Polymorphism)来创建一个抽象的`Shape`类,然后分别创建`Rectangle`和`Circle`类作为具体的形状实现。这里是一个简单的示例:
```java
// 抽象Shape类
public abstract class Shape {
// 公共的方法,用于计算周长和面积
public abstract double getPerimeter();
public abstract double getArea();
}
// 具体的图形类 - 长方形
public class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
public double getPerimeter() {
return 2 * (width + height);
}
@Override
public double getArea() {
return width * height;
}
}
// 具体的图形类 - 圆形
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double getPerimeter() {
return 2 * Math.PI * radius;
}
@Override
public double getArea() {
return Math.PI * Math.pow(radius, 2);
}
}
// 主函数,展示多态的应用
public static void main(String[] args) {
Shape rectangle = new Rectangle(5, 3);
Shape circle = new Circle(4);
System.out.println("Rectangle perimeter: " + rectangle.getPerimeter());
System.out.println("Rectangle area: " + rectangle.getArea());
System.out.println("Circle perimeter: " + circle.getPerimeter());
System.out.println("Circle area: " + circle.getArea());
}
```
在这个例子中,`Shape`是抽象的父类,`Rectangle`和`Circle`都是其具体子类。通过将形状实例化为`Shape`类型的引用,我们可以在主函数中统一处理各种形状的周长和面积计算,这就是多态的体现。
阅读全文
相关推荐
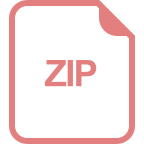
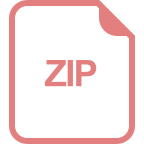
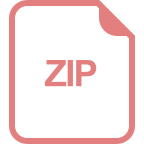
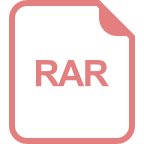
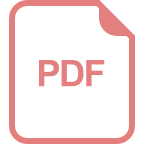
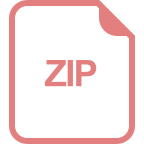
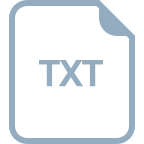
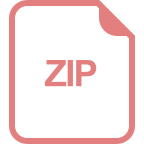
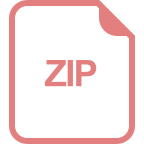
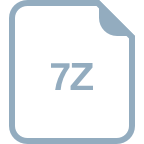
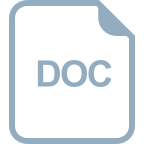
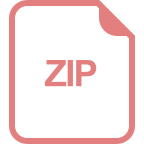
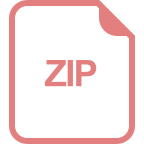
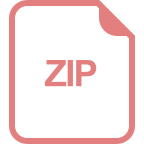
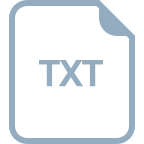
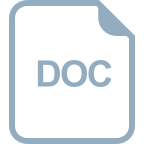
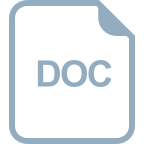
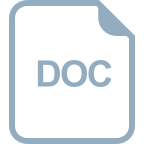