springboot+mybatisplus session
时间: 2023-08-05 10:02:30 浏览: 59
在 Spring Boot 中使用 MyBatis Plus,你可以使用 MyBatis Plus 提供的 Session API 进行数据库操作。下面是一个简单的示例:
首先,确保在你的项目中添加了 Spring Boot 和 MyBatis Plus 的依赖。
在你的 Spring Boot 配置文件中,配置数据库连接信息,例如:
```
spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase
spring.datasource.username=username
spring.datasource.password=password
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
```
创建一个实体类,用于映射数据库表的字段。可以使用 `@TableName` 注解来指定表名,使用 `@TableId` 注解来指定主键。
```java
import com.baomidou.mybatisplus.annotation.*;
import lombok.Data;
@Data
@TableName("user")
public class User {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
private Integer age;
@TableField(fill = FieldFill.INSERT)
private LocalDateTime createTime;
@TableField(fill = FieldFill.INSERT_UPDATE)
private LocalDateTime updateTime;
@Version
private Integer version;
@TableLogic
private Integer deleted;
}
```
创建一个 Mapper 接口,继承自 `BaseMapper`,用于定义数据库操作的方法。
```java
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface UserMapper extends BaseMapper<User> {
}
```
在 Service 层中,注入 `UserMapper`,并使用 `UserMapper` 的方法进行数据库操作。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
private final UserMapper userMapper;
@Autowired
public UserService(UserMapper userMapper) {
this.userMapper = userMapper;
}
public User getUserById(Long id) {
return userMapper.selectById(id);
}
// 其他数据库操作方法...
}
```
这样,你就可以在 Spring Boot 中使用 MyBatis Plus 进行数据库操作了。你可以在 Service 层调用相应的方法,通过 `UserMapper` 对数据库进行增删改查操作。
希望这能帮到你!如果有任何问题,请随时提问。
相关推荐
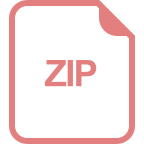
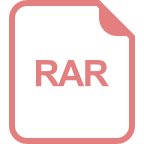
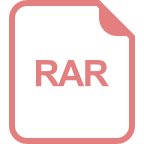














