用c语言编写 图书馆管理系统中的恢复,备份功能的代码
时间: 2024-09-19 17:04:07 浏览: 43
在C语言中,图书馆管理系统的恢复和备份功能通常涉及到文件操作和数据结构的处理。由于实际的代码长度较长,这里提供一个简化版的概念性示例,展示了如何基本地实现数据库备份和恢复的基本流程:
```c
#include <stdio.h>
#include <stdlib.h>
// 模拟图书数据结构
typedef struct {
char book_id[20];
char title[50];
char author[50];
} Book;
// 恢复函数 (假设从一个文本文件中读取)
void restore_database(char *filename) {
FILE *file = fopen(filename, "r");
if (file == NULL) {
printf("Failed to open file for reading.\n");
return;
}
Book restored_books[100]; // 假设最多100本书
int count = fread(restored_books, sizeof(Book), 100, file);
fclose(file);
// 然后遍历restored_books并添加到数据库中
for (int i = 0; i < count; i++) {
// 在实际项目中,你需要连接数据库并执行相应的插入操作
printf("Restored book: %s - %s by %s\n", restored_books[i].book_id, restored_books[i].title, restored_books[i].author);
}
}
// 备份函数 (假设保存到一个文本文件中)
void backup_database(char *filename, Book *books, int num_books) {
FILE *file = fopen(filename, "w");
if (file == NULL) {
printf("Failed to open file for writing.\n");
return;
}
for (int i = 0; i < num_books; i++) {
fprintf(file, "%s\t%s\t%s\n", books[i].book_id, books[i].title, books[i].author);
}
fclose(file);
printf("Backup completed.\n");
}
int main() {
// 这里只是模拟,实际应用需要连接数据库获取或更新数据
Book library[] = { /* ... */ };
backup_database("library_backup.txt", library, sizeof(library) / sizeof(library[0]));
// 假如数据丢失或系统故障,可以尝试恢复
restore_database("library_backup.txt");
return 0;
}
```
阅读全文
相关推荐
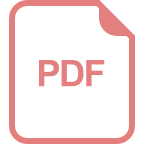
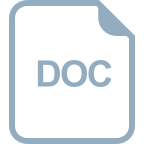
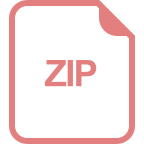
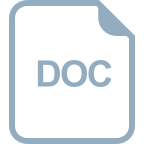

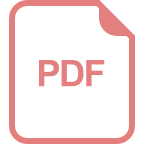
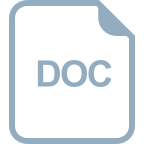
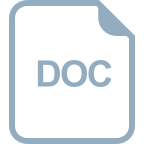
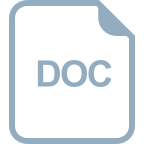
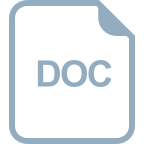
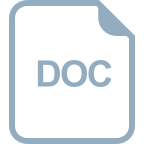
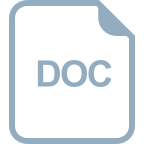
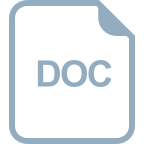
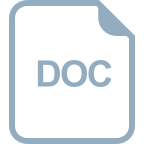
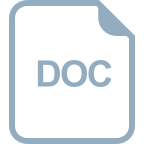
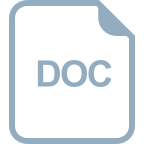


