c语言 计算学生平均成绩,计算各科成绩最高分
时间: 2024-06-14 10:05:14 浏览: 30
以下是计算学生平均成绩和各科成绩最高分的C语言代码示例:
```c
#include <stdio.h>
struct Student {
int id;
char name[50];
char className[50];
float mathScore;
float englishScore;
float computerScore;
};
int main() {
struct Student students[100];
int numStudents;
// 从用户输入获取学生信息
printf("请输入学生人数:");
scanf("%d", &numStudents);
for (int i = 0; i < numStudents; i++) {
printf("请输入第%d个学生的学号:", i + 1);
scanf("%d", &students[i].id);
printf("请输入第%d个学生的姓名:", i + 1);
scanf("%s", students[i].name);
printf("请输入第%d个学生的班级:", i + 1);
scanf("%s", students[i].className);
printf("请输入第%d个学生的数学成绩:", i + 1);
scanf("%f", &students[i].mathScore);
printf("请输入第%d个学生的英语成绩:", i + 1);
scanf("%f", &students[i].englishScore);
printf("请输入第%d个学生的计算机成绩:", i + 1);
scanf("%f", &students[i].computerScore);
}
// 计算每个学生的平均成绩
for (int i = 0; i < numStudents; i++) {
float averageScore = (students[i].mathScore + students[i].englishScore + students[i].computerScore) / 3;
printf("学生%d的平均成绩为:%.2f\n", students[i].id, averageScore);
}
// 计算各科成绩的最高分
float maxMathScore = students[0].mathScore;
float maxEnglishScore = students[0].englishScore;
float maxComputerScore = students[0].computerScore;
for (int i = 1; i < numStudents; i++) {
if (students[i].mathScore > maxMathScore) {
maxMathScore = students[i].mathScore;
}
if (students[i].englishScore > maxEnglishScore) {
maxEnglishScore = students[i].englishScore;
}
if (students[i].computerScore > maxComputerScore) {
maxComputerScore = students[i].computerScore;
}
}
printf("数学成绩的最高分为:%.2f\n", maxMathScore);
printf("英语成绩的最高分为:%.2f\n", maxEnglishScore);
printf("计算机成绩的最高分为:%.2f\n", maxComputerScore);
return 0;
}
```
相关推荐
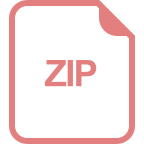
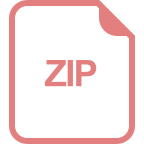
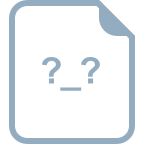














