visual studio中取绝对值函数怎么用
时间: 2023-12-17 20:03:17 浏览: 103
在 Visual Studio 中,你可以使用 **abs()** 函数来求一个数的绝对值。例如,如果你想求 -5 的绝对值,你可以使用以下代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main()
{
float n = -5;
cout << "|-5| = " << abs(n) << endl;
return 0;
}
```
相关问题
visual studio中生成函数
在Visual Studio中,你可以使用三个连续的反斜杠///来生成函数说明文档的模板。这个功能非常简单,只需要在你自己写的函数的函数头上方输入这个注释符号即可。Visual Studio会立即为你生成一个函数说明文档的模板。这个模板包括了函数的参数、返回值和函数的用途等信息,帮助其他开发人员更好地理解和使用你的函数。通过生成函数说明文档模板,你可以使你的代码更具可读性和可维护性,提高团队协作效率。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [Visual Studio2022快速生成函数说明文档](https://blog.csdn.net/strive_max/article/details/128052899)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *3* [Visual Studio中MATLAB和.Net之间的数据转换:本示例说明如何在Visual Studio中为MATLAB函数导入或导出不同...](https://download.csdn.net/download/weixin_38647039/19196418)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
怎么在Visual Studio软件中使用strcmp 函数
strcmp 函数是C/C++语言中用于比较两个字符串的函数,可以在Visual Studio软件中使用。下面是使用strcmp函数的示例代码:
```c++
#include <stdio.h>
#include <string.h>
int main()
{
char str1[20] = "Hello";
char str2[20] = "World";
if (strcmp(str1, str2) == 0)
printf("两个字符串相等\n");
else
printf("两个字符串不相等\n");
return 0;
}
```
在上面的代码中,首先包含了头文件`<stdio.h>`和`<string.h>`。然后声明了两个字符串数组`str1`和`str2`,并初始化为"Hello"和"World"。接着使用`strcmp`函数比较两个字符串,如果相等,则输出"两个字符串相等",否则输出"两个字符串不相等"。
需要注意的是,`strcmp`函数会比较两个字符串的每一个字符,直到找到不同的字符或者遇到字符串结束符'\0'为止。如果两个字符串相等,则返回值为0;如果第一个字符串大于第二个字符串,则返回值为正数;如果第一个字符串小于第二个字符串,则返回值为负数。
相关推荐
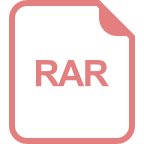
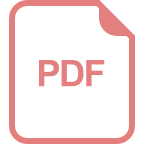
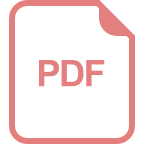












