如何用C语言构建一个N-S(流程)图来确定任意一年中一个月的特定日期是该月的第几天?
时间: 2024-11-23 17:41:23 浏览: 12
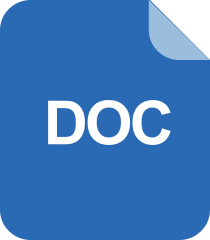
精品(2021-2022年)资料计算机图形学考试题.doc
在C语言中,你可以使用结构体和循环来构建一个简单的N-S(流程)图来计算任意年份和月份中的特定日期是该月的第几天。这里是一个基本步骤的例子:
1. 首先,创建一个表示月份的结构体,包含月份的名称和天数数组,因为每个月的天数可能会有所不同(比如二月有28或29天,闰年时为29天)。
```c
typedef struct {
char name[10];
int days[];
} Month;
```
2. 初始化Month结构体,例如,存储所有标准月份的天数。
```c
Month months[] = {
{"January", {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}},
// ... 其他月份...
};
```
3. 定义一个函数来接收年份、月份和日期作为输入,并通过查找结构体内的天数组来计算指定日期在该月的位置。
```c
int day_in_month(int year, int month, int date) {
if (month < 1 || month > 12 || date < 1 || date > months[month - 1].days[date - 1]) {
return -1; // 返回错误,如果输入无效
}
// 如果是闰年且2月,需要检查是否是闰年
if (month == 2 && is_leap_year(year)) {
return date;
} else {
return date - 1; // 直接返回,非闰年的2月和其他月份都是从第一天开始计数
}
}
// 判断是否为闰年(这是一个简化版本,实际应考虑世纪闰年规则)
bool is_leap_year(int year) {
return year % 4 == 0 && (year % 100 != 0 || year % 400 == 0);
}
```
4. 使用此函数并提供用户输入的年份、月份和日期来获取结果。
```c
int main() {
int year, month, date;
printf("Enter the year, month (1-12), and date: ");
scanf("%d %d %d", &year, &month, &date);
int result = day_in_month(year, month, date);
if (result >= 0) {
printf("The date %d is the %dth day of %s.\n", date, result + 1, months[month - 1].name);
} else {
printf("Invalid input.\n");
}
return 0;
}
```
阅读全文
相关推荐
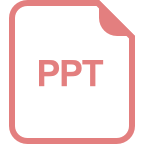
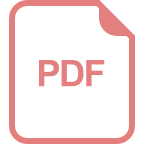
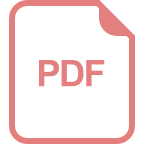
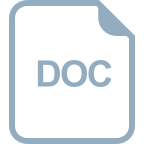
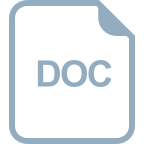
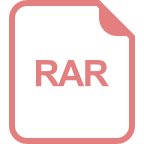
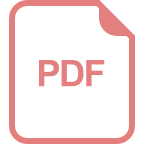
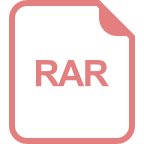
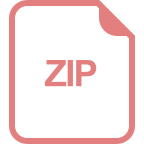
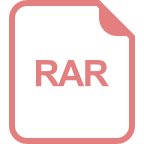
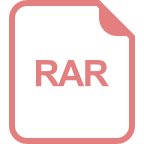
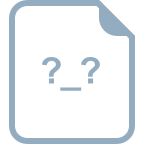
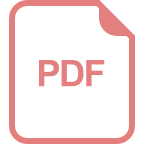
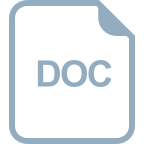
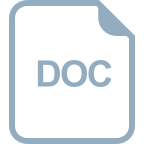
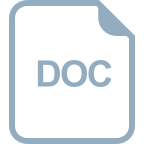