编写一个秒表类StopWatch,成员变量和方法自定。编写一个支持快速排序算法的类QuickSort,成员变量和方法自定。对100000, 1000000, …等不同长度的数组中的元素(元素的值随机给定)进行排序,并用秒表对象来记录排序所用的时间。
时间: 2024-10-23 08:16:54 浏览: 22
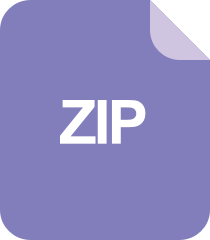
go-stopwatch:一个简单的秒表来测量、获取和记录经过的时间
首先,我们可以定义两个类,一个是StopWatch类,用于计时,另一个是QuickSort类,负责快速排序算法。
**StopWatch 类**
```java
public class StopWatch {
private long startTime;
// 构造函数开始计时
public StopWatch() {
this.startTime = System.nanoTime();
}
// 记录并返回停止时间
public long stopAndReturnTime() {
return System.nanoTime() - startTime;
}
// 获取当前运行时间(单位纳秒)
public double getCurrentTime() {
return (double) (System.nanoTime() - startTime) / 1e9; // 转换为秒
}
}
```
**QuickSort 类**
```java
import java.util.Arrays;
import java.util.Random;
public class QuickSort {
public void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
private int partition(int[] arr, int low, int high) {
int pivotValue = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] <= pivotValue) {
i++;
swap(arr, i, j);
}
}
swap(arr, i + 1, high); // 将pivot放到正确的位置
return i + 1;
}
private void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
```
然后,我们可以在主程序中创建StopWatch和QuickSort实例,并测试排序:
```java
public static void main(String[] args) {
Random rand = new Random();
for (int size : Arrays.asList(100000, 500000, 1000000)) {
int[] arr = new int[size];
for (int i = 0; i < size; i++) {
arr[i] = rand.nextInt(size * 10);
}
StopWatch stopwatch = new StopWatch();
QuickSort quickSortInstance = new QuickSort();
quickSortInstance.quickSort(arr, 0, arr.length - 1);
long elapsedTime = stopwatch.stopAndReturnTime();
System.out.printf("Sorted array of length %d in %.6f seconds.\n", size, elapsedTime);
}
}
```
阅读全文
相关推荐
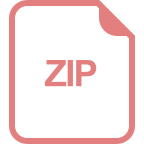
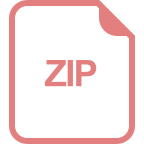
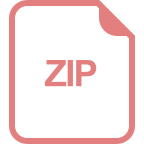
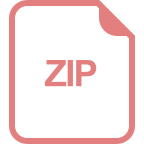
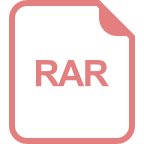
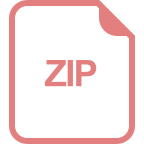
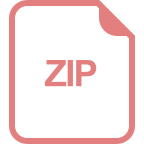
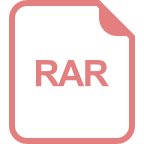
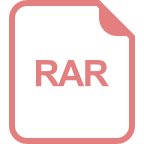
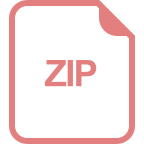
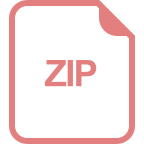
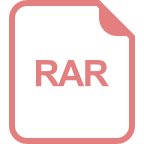
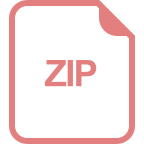
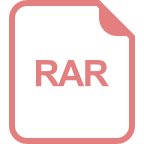

