matlab 判断数组中是否有相同的元素
时间: 2024-09-15 14:08:52 浏览: 275
在MATLAB中,判断数组中是否有相同元素可以使用`ismember()`函数或者直接通过循环和条件语句来实现。以下是两种常用方法:
1. 使用`ismember()`函数:
```matlab
% 假设数组A是一个一维或二维数组
A = [1 2 3; 4 5 6];
% 检查是否存在重复
isDuplicate = any(ismember(A(:), A(:)));
```
`ismember()`会返回一个布尔矩阵,如果存在重复元素,则至少有一个true值。
2. 直接循环检查:
```matlab
A = [1 2 3; 4 5 6];
duplicateFound = false;
% 遍历每个元素与数组中的其他元素比较
for i = 1:numel(A)
for j = (i+1):numel(A)
if A(i) == A(j)
duplicateFound = true;
break;
end
end
if duplicateFound
break; % 如果找到重复就停止内层循环
end
end
```
这里假设`numel()`返回数组的元素数目。
相关问题
matlab判断数组中重复元素
可以使用unique函数和isequal函数来判断数组中是否有重复元素。
方法一:
使用unique函数将数组去重,然后比较原数组和去重后的数组是否相等。
示例代码:
a = [1, 2, 3, 4, 5, 1];
b = unique(a);
if isequal(a, b)
disp('没有重复元素');
else
disp('有重复元素');
end
方法二:
使用ismember函数判断数组中是否有重复元素。
示例代码:
a = [1, 2, 3, 4, 5, 1];
if length(unique(a)) == length(a)
disp('没有重复元素');
else
disp('有重复元素');
end
matlab判断数组中是否存在某个值
在MATLAB中,你可以使用`exist`函数或者`isequal`、`any`或`all`函数以及逻辑运算符来判断数组中是否包含某个特定值。以下是几种常用的方法:
1. **exist**函数:它用于检查变量是否存在以及其类型。例如,如果你有一个名为`array`的数组,可以这样做:
```matlab
value = some_value; % 替换为你要查找的值
if exist('array', 'member', value)
disp('Value exists in the array');
else
disp('Value does not exist in the array');
end
```
2. **isequal**与**any/all**:如果想要确认数组中有任何一个元素等于目标值,则可以使用`any(array == value)`,如果所有元素都相等则用`all(array == value)`。例如:
```matlab
if any(array == value)
disp('Value exists in the array');
else
disp('Value does not exist in the array');
end
```
3. **逻辑运算符**:直接比较数组和值,例如 `any(array == value)` 或者 `~(array == value)`, 如果返回`true`表示存在。
阅读全文
相关推荐
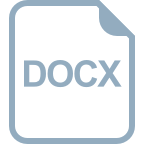
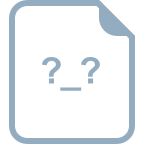
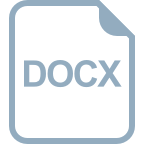













