go语言 pbe加密算法实现
时间: 2024-01-30 09:02:40 浏览: 240
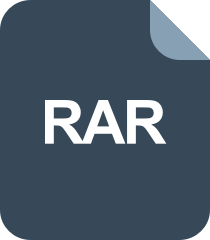
PBE 加密实例
以下是使用Go语言实现PBE加密算法的示例代码:
```go
package main
import (
"crypto/cipher"
"crypto/des"
"crypto/md5"
"encoding/base64"
"fmt"
)
func main() {
// 设置加密参数
password := "123456" // 密码
salt := "salt" // 盐值
iterations := 1000 // 迭代次数
// 生成密钥
key := pbkdf1([]byte(password), []byte(salt), iterations, 8)
// 加密明文
plaintext := "hello world"
ciphertext, err := pbeEncrypt([]byte(plaintext), key)
if err != nil {
panic(err)
}
// 输出加密结果
fmt.Println(base64.StdEncoding.EncodeToString(ciphertext))
// 解密密文
decrypted, err := pbeDecrypt(ciphertext, key)
if err != nil {
panic(err)
}
// 输出解密结果
fmt.Println(string(decrypted))
}
// PBE加密
func pbeEncrypt(plaintext []byte, key []byte) ([]byte, error) {
// 创建加密器
block, err := des.NewCipher(key)
if err != nil {
return nil, err
}
iv := make([]byte, block.BlockSize())
stream := cipher.NewCTR(block, iv)
// 加密明文
ciphertext := make([]byte, len(plaintext))
stream.XORKeyStream(ciphertext, plaintext)
return ciphertext, nil
}
// PBE解密
func pbeDecrypt(ciphertext []byte, key []byte) ([]byte, error) {
// 创建解密器
block, err := des.NewCipher(key)
if err != nil {
return nil, err
}
iv := make([]byte, block.BlockSize())
stream := cipher.NewCTR(block, iv)
// 解密密文
plaintext := make([]byte, len(ciphertext))
stream.XORKeyStream(plaintext, ciphertext)
return plaintext, nil
}
// PBKDF1算法
func pbkdf1(password []byte, salt []byte, iterations int, keyLen int) []byte {
key := password
for i := 0; i < iterations; i++ {
data := append(key, salt...)
hash := md5.Sum(data)
key = hash[:]
}
return key[:keyLen]
}
```
阅读全文
相关推荐
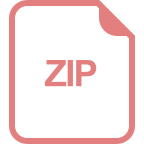
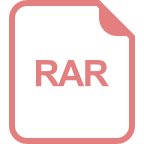
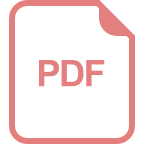
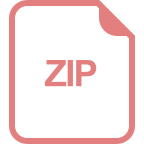
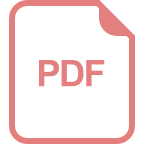
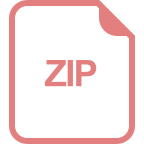
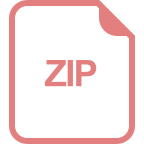
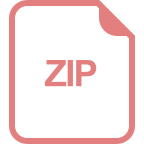
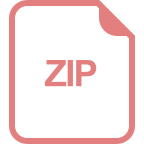

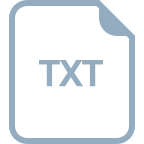
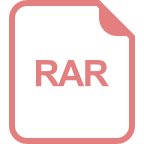
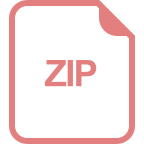
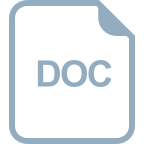
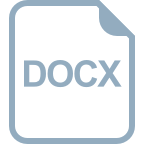
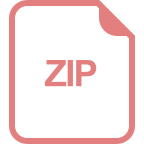