Java复制一个文件夹的文件到另一个文件夹下,当文件大于5个时,每5个文件复制一次的示例代码
时间: 2024-09-27 13:10:51 浏览: 23
在Java中,你可以使用`java.io.File`类以及`Files.copy()`方法来复制文件。这里是一个简单的示例,展示了如何按照每五个文件一组的方式复制文件。这个示例假设你已经有了源目录、目标目录,以及一个文件列表:
```java
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class CopyFolderWithBatch {
private static final int BATCH_SIZE = 5; // 每批复制5个文件
public static void copyFilesInBatches(String srcDir, String destDir) throws IOException {
File srcFile = new File(srcDir);
if (!srcFile.exists() || !srcFile.isDirectory()) {
throw new IllegalArgumentException("Source directory does not exist or is not a directory");
}
File destFile = new File(destDir);
if (!destFile.exists()) {
destFile.mkdirs(); // 创建目标目录如果不存在
}
List<File> filesToCopy = new ArrayList<>();
for (File file : srcFile.listFiles()) {
if (file.isFile()) {
filesToCopy.add(file);
}
}
int batchSize = 0;
for (File file : filesToCopy) {
Path srcPath = Paths.get(file.getAbsolutePath());
Path destPath = Paths.get(destDir + '/' + file.getName());
try (InputStream in = Files.newInputStream(srcPath);
OutputStream out = Files.newOutputStream(destPath)) {
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
System.out.println("Copied " + file.getName() + " to " + destPath);
} catch (IOException e) {
System.err.println("Error copying file " + file.getName());
e.printStackTrace();
}
batchSize++;
if (batchSize == BATCH_SIZE) {
System.out.println("Copied batch of " + BATCH_SIZE + " files.");
batchSize = 0;
}
}
if (batchSize > 0) {
System.out.println("Copied remaining " + batchSize + " files.");
}
}
public static void main(String[] args) {
try {
copyFilesInBatches("/path/to/source/folder", "/path/to/destination/folder");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
注意:将`"/path/to/source/folder"`和`"/path/to/destination/folder"`替换为你实际的源目录和目标目录。
阅读全文
相关推荐
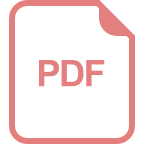
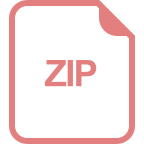
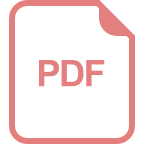


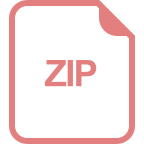
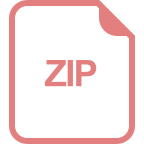
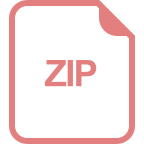
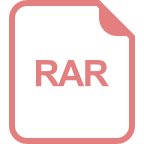
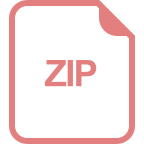
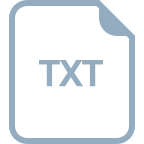
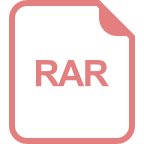
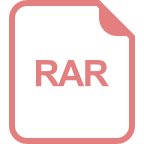
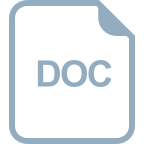


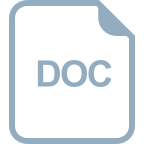

