高德地图轨迹展示&巡航,巡航结束事件
时间: 2023-11-25 21:44:53 浏览: 58
要在高德地图上展示轨迹并实现巡航效果,可以使用 Vue.js 结合高德地图 JavaScript API。下面是一个简单的示例代码:
```html
<template>
<div>
<div id="map" style="width: 800px; height: 600px;"></div>
</div>
</template>
<script>
export default {
mounted() {
// 创建地图
const map = new AMap.Map('map', {
zoom: 15,
center: [116.39, 39.9]
});
// 定义轨迹路径
const path = [
[116.39, 39.9],
[116.4, 39.91],
[116.41, 39.92],
// ...
];
// 创建轨迹线
const polyline = new AMap.Polyline({
map: map,
path: path,
strokeColor: '#3366FF',
strokeOpacity: 1,
strokeWeight: 3
});
// 创建小车标记
const marker = new AMap.Marker({
map: map,
icon: new AMap.Icon({
size: new AMap.Size(50, 50),
image: 'https://webapi.amap.com/images/car.png',
imageSize: new AMap.Size(50, 50)
})
});
// 设置小车标记的位置和角度
function setMarkerPosition(position) {
marker.setPosition(position);
marker.setAngle(getAngle(position));
}
// 获取小车标记的角度
function getAngle(position) {
// 根据当前位置和下一个位置计算角度
// ...
}
// 小车巡航的动画
function cruise() {
let index = 0;
const timer = setInterval(() => {
setMarkerPosition(path[index]);
index++;
if (index >= path.length) {
clearInterval(timer);
// 触发巡航结束事件
this.$emit('cruiseFinish');
}
}, 1000);
}
// 开始小车巡航
cruise();
}
}
</script>
```
在上述代码中,使用 AMap.Polyline 创建轨迹线,并使用 AMap.Marker 创建小车标记。通过设置小车标记的位置和角度实现小车在轨迹上的移动效果。当巡航结束时,通过 `$emit` 方法触发 `cruiseFinish` 事件。
在父组件中,可以监听 `cruiseFinish` 事件并执行相应的操作。例如:
```html
<template>
<div>
<my-map @cruiseFinish="handleCruiseFinish"></my-map>
</div>
</template>
<script>
import MyMap from './MyMap.vue';
export default {
components: {
MyMap
},
methods: {
handleCruiseFinish() {
console.log('巡航结束');
// 执行巡航结束后的操作
}
}
}
</script>
```
在父组件中,通过监听 `cruiseFinish` 事件可以捕获巡航结束的时机,并执行相应的操作。
相关推荐
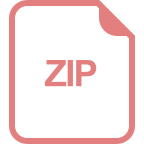
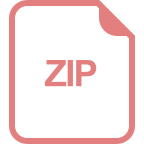














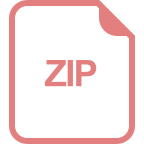