关于分配任务的一个c语言程序
时间: 2024-09-24 19:02:08 浏览: 31
在C语言中,编写一个简单的任务分配程序可能会涉及到线程和任务队列的概念。这里我们可以用一个基本的多线程模型来实现任务的分配,每个线程代表一个任务接收者,而任务则放入一个共享的任务队列中。下面是一个简化的示例,使用POSIX线程库(pthread):
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define MAX_THREADS 5
#define TASKS 10
typedef struct {
int id;
char* task;
} Task;
// 创建任务队列
Task queue[TASKS] = { ... }; // 初始化任务列表
int tasks_left = TASKS;
void *task_worker(void *arg) {
Task *task = (Task*) arg;
printf("Thread %d received task: %s\n", task->id, task->task);
free(task); // 释放任务空间
tasks_left--;
if (tasks_left == 0) {
pthread_exit(NULL);
}
}
int main() {
pthread_t threads[MAX_THREADS];
// 分配任务给各个线程
for (int i = 0; i < MAX_THREADS && tasks_left > 0; i++) {
Task task = queue[i];
pthread_create(&threads[i], NULL, task_worker, &task);
// 简单同步,等待所有线程开始工作
while (tasks_left > 0 && pthread_equal(pthread_self(), threads[i])) {
sleep(1);
}
}
// 等待所有线程完成
for (int i = 0; i < MAX_THREADS; i++) {
pthread_join(threads[i], NULL);
}
return 0;
}
阅读全文
相关推荐



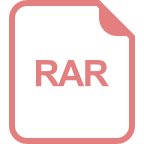
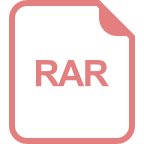






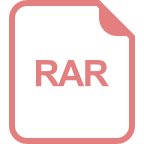

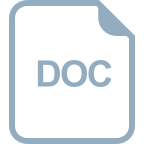
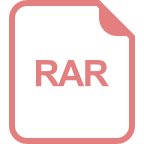


