vue2实现无缝轮播
时间: 2023-10-20 08:29:24 浏览: 142
在 Vue 2 中实现无缝轮播可以通过以下步骤进行:
1. 创建一个 Vue 组件,命名为`Carousel`。
2. 在`Carousel`组件中,定义一个数组来存储需要轮播的图片列表。
3. 在`mounted`生命周期钩子函数中,初始化轮播的一些参数,例如当前显示的图片索引、定时器等。
4. 在模板中,使用`v-for`指令循环渲染图片列表,并设置每个图片项的样式,使其水平排列。
5. 使用`transform: translateX()`样式属性,对轮播项进行水平移动,实现轮播效果。可以通过计算当前索引和图片宽度来设置移动的距离。
6. 添加一个定时器,在一定时间间隔内更新当前显示的图片索引,实现自动轮播效果。
7. 监听鼠标滑入和滑出事件,在鼠标滑入时清除定时器,在鼠标滑出时重新启动定时器,实现鼠标悬停时暂停轮播的效果。
以下是一个简单示例代码:
```html
<template>
<div class="carousel">
<div class="carousel-wrapper" :style="{ transform: `translateX(${translateX}px)` }">
<div v-for="(item, index) in images" :key="index" class="carousel-item">
<img :src="item" alt="carousel item" />
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
images: [
'image1.jpg',
'image2.jpg',
'image3.jpg',
'image4.jpg',
],
currentIndex: 0,
translateX: 0,
intervalId: null,
};
},
mounted() {
this.startCarousel();
},
beforeDestroy() {
clearInterval(this.intervalId);
},
methods: {
startCarousel() {
this.intervalId = setInterval(() => {
this.nextSlide();
}, 3000);
},
nextSlide() {
if (this.currentIndex === this.images.length - 1) {
this.currentIndex = 0;
this.translateX = 0;
} else {
this.currentIndex++;
this.translateX -= this.$refs.carouselItem.offsetWidth;
}
},
},
};
</script>
<style>
.carousel {
width: 100%;
overflow: hidden;
}
.carousel-wrapper {
display: flex;
transition: transform 0.5s;
}
.carousel-item {
flex-shrink: 0;
}
</style>
```
以上代码是一个简单的示例,实现了简单的无缝轮播效果。你可以根据需求进行进一步的样式和功能定制。
阅读全文
相关推荐
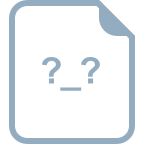
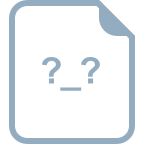
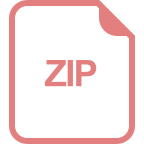
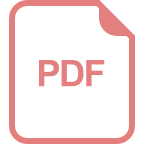
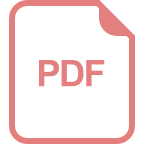
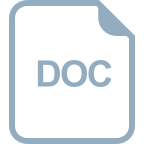
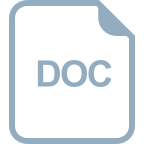
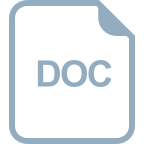
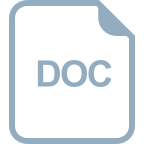
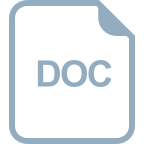




