如何在React 16.0.0中实现组件的状态管理和生命周期控制?请结合Hooks和类组件给出示例。
时间: 2024-11-01 07:12:00 浏览: 20
在React 16.0.0版本中,状态管理和生命周期控制可以通过类组件的传统方式,以及新引入的Hooks API来实现。首先,我们来看看如何在类组件中管理状态和生命周期。
参考资源链接:[Fullstack React指南:深度解析ReactJS及其生态系统](https://wenku.csdn.net/doc/2semozqjri?spm=1055.2569.3001.10343)
对于类组件,状态通常是通过构造函数(constructor)中的state对象来初始化,然后通过setState()方法进行更新。而生命周期方法则定义了组件从创建到挂载,再到更新和卸载的整个过程。例如:
```***
***ponent {
constructor(props) {
super(props);
this.state = { count: 0 };
}
componentDidMount() {
console.log('Component mounted.');
}
componentDidUpdate() {
console.log('Component updated.');
}
componentWillUnmount() {
console.log('Component will unmount.');
}
render() {
return (
<div>
<p>You clicked {this.state.count} times</p>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>
Click me
</button>
</div>
);
}
}
```
接下来,我们看看如何使用Hooks来完成同样的任务。Hooks是React 16.8中引入的一个新特性,它允许开发者在不编写类的情况下使用state和其他React特性。useState用于状态管理,useEffect可以处理组件生命周期相关的逻辑。
```jsx
import React, { useState, useEffect } from 'react';
function HookCounter() {
const [count, setCount] = useState(0);
useEffect(() => {
console.log('Component did mount and update.');
return () => console.log('Component will unmount.');
}, []); // 空数组确保useEffect只运行一次
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
```
在这个Hooks版本的计数器组件中,useState直接在函数组件内部声明了count状态变量和修改它的setCount函数。而useEffect则模拟了componentDidMount和componentDidUpdate生命周期方法的行为。如果需要模拟componentWillUnmount的行为,可以在useEffect的返回函数中添加清理代码。
通过这些示例,可以看出类组件和Hooks各有优劣。类组件提供了更丰富的生命周期方法,而Hooks则使代码更加简洁,尤其在函数组件中可以更好地组合和重用逻辑。《Fullstack React指南:深度解析ReactJS及其生态系统》一书详细讨论了这些主题,并提供了更多实用的技术细节和实战案例。
参考资源链接:[Fullstack React指南:深度解析ReactJS及其生态系统](https://wenku.csdn.net/doc/2semozqjri?spm=1055.2569.3001.10343)
阅读全文
相关推荐
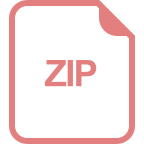
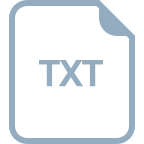
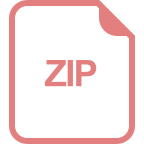
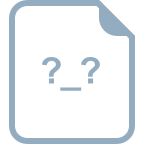
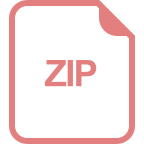
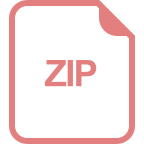
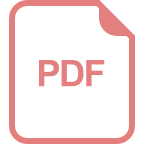
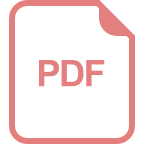
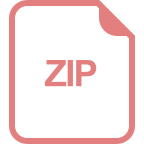
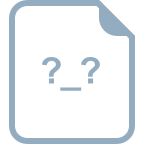
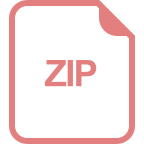
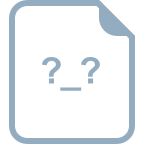
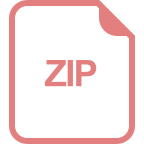
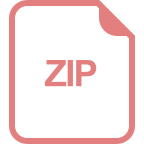
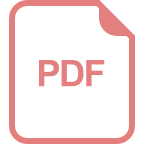
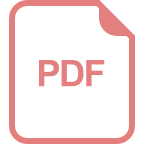
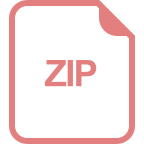
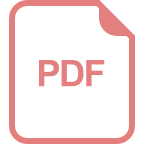