import axios from "axios"; import router from "@/router"; import { userStore } from "@/store/module/user"; import { message } from "ant-design-vue"; import { loadingStore } from "@/store/module/utils"; import { t } from "./util"; const service = axios.create({ timeout: 60000, headers: { "Content-type": "application/json;charset=utf-8" }, }); service.interceptors.request.use( (config: any) => { const user = userStore(); if (user.getToken) { config.headers["Authorization"] = user.getToken; } return config; }, () => { const loading = loadingStore(); loading.setLoading(false); } ); service.interceptors.response.use( (config) => { const user = userStore(); const { code } = config.data; if ([10002, 10003, 10011].includes(code)) { user.ClearToken(); router.push({ path: "/login" }); window.location.reload(); } else { if (code !== 0 && code) { const current = user.errorCode.find((el) => el.code === code); if (current) { message.error(current?.info); } else { message.error(t("common.unkError")); } } } return config; }, (error) => { const user = userStore(); const loading = loadingStore(); if ([404, 500].includes(error.response.status)) user.LoginOut(); window.location.reload(); loading.setLoading(false); } ); export default service; 详细解释段代码
时间: 2024-04-21 08:22:24 浏览: 125
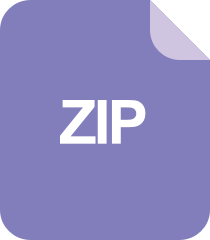
Error:in ./node-modules/axios/lib/platform/index.js
这段代码是一个封装了axios的HTTP请求库。让我们一步一步详细解释一下。
首先,这段代码引入了一些依赖,包括axios、router、userStore、message和loadingStore等。axios是一个用于发送HTTP请求的库,router用于进行路由导航,userStore和loadingStore是自定义的store模块,message是用于显示提示信息的库。
然后,通过axios.create()创建了一个axios实例service,并设置了超时时间为60秒,以及请求头的Content-type为application/json;charset=utf-8。
接下来,通过service.interceptors.request.use()添加了一个请求拦截器。该拦截器会在每次请求发送之前被调用。在拦截器中,首先获取userStore中的用户信息,如果存在token,则将其添加到请求头的Authorization字段中。然后返回配置对象config。
如果请求发生错误,拦截器会调用service.interceptors.request.use()方法的第二个回调函数。在这个回调函数中,首先获取loadingStore中的loading状态,并将其设置为false。
接下来,通过service.interceptors.response.use()添加了一个响应拦截器。该拦截器会在每次收到响应后被调用。在拦截器中,首先获取userStore中的用户信息和响应数据中的code字段。
如果code的值等于10002、10003或10011,则表示token无效或过期,需要清除用户信息,并进行页面跳转至登录页面。然后使用window.location.reload()重新加载页面。
如果code的值不等于0并且存在,则根据code在userStore中的errorCode数组中查找对应的错误信息,并使用message.error()显示错误信息。如果在errorCode数组中找不到对应的错误信息,则显示一个通用的未知错误信息。
最后,通过service.interceptors.response.use()方法的第二个回调函数处理响应错误。在这个回调函数中,首先获取userStore中的用户信息和loadingStore中的loading状态。如果响应状态码为404或500,则表示请求发生了错误,需要清除用户信息并重新加载页面。然后将loading状态设置为false。
最后,通过export default将service导出为默认模块。这样,在其他文件中引入该模块时,可以直接使用该实例来发送HTTP请求。
阅读全文
相关推荐
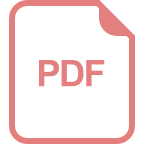
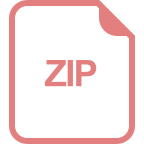



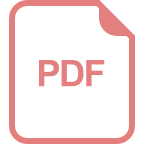
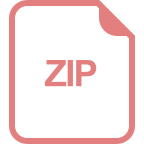
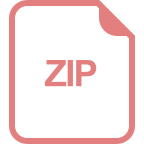









