跌到检测 yolov7
时间: 2023-09-13 22:11:54 浏览: 48
你好!要检测 YOLOv7 的对象,需要进行一些步骤。首先,确保你已经安装了必要的库和环境,如 PyTorch、OpenCV 等。然后,你可以按照以下步骤进行检测:
1. 下载 YOLOv7 的权重文件和配置文件。你可以在 Darknet 官方网站上找到这些文件。
2. 加载模型:
```python
import torch
from models import Darknet
model = Darknet('path/to/config_file.cfg')
model.load_state_dict(torch.load('path/to/weights_file.weights'))
model.eval()
```
3. 对图像进行预处理:
```python
import cv2
import numpy as np
def preprocess_image(image_path):
image = cv2.imread(image_path)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image = cv2.resize(image, (model.width, model.height))
image = np.transpose(image / 255., (2, 0, 1))
image = np.expand_dims(image, 0)
return torch.from_numpy(image).float()
image = preprocess_image('path/to/image.jpg')
```
4. 进行对象检测:
```python
with torch.no_grad():
detections = model(image)
```
检测结果将包含在 `detections` 变量中。
5. 解析检测结果:
```python
from utils import non_max_suppression, load_classes
classes = load_classes('path/to/class_labels.txt')
detections = non_max_suppression(detections, conf_threshold, nms_threshold)
for detection in detections:
x1, y1, x2, y2, conf, cls_conf, cls_pred = detection
class_label = classes[int(cls_pred)]
print(f'Object: {class_label}, Confidence: {conf}')
```
这将输出检测到的对象及其置信度。
希望这些步骤能帮助到你进行 YOLOv7 的对象检测!
相关推荐
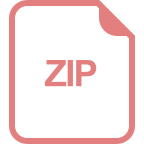
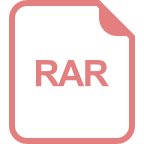















