从提供的视频数据中提取相关信息,包括每个观测点在特定时间段内通过的车辆数、车流速度等。为便于分析,假设我们每分钟提取一次数据。python代码
时间: 2024-09-22 16:02:34 浏览: 25
在Python中,处理视频数据并从中提取车辆信息通常涉及到图像处理和计算机视觉技术,如OpenCV库。以下是一个简化的步骤概述,以及如何编写一段基本的代码来实现这个过程:
1. **安装必要的库**:
首先需要安装`opencv-python`, `numpy`, 和 `matplotlib` 等库,可以使用`pip`命令安装:
```
pip install opencv-python numpy matplotlib
```
2. **读取和预处理视频**:
使用`cv2.VideoCapture`读取视频文件,并对每一帧进行灰度化和缩放操作以提高处理效率:
```python
import cv2
cap = cv2.VideoCapture('video.mp4')
frame_width = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
frame_height = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
while True:
ret, frame = cap.read()
if not ret:
break
gray_frame = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 对帧进行缩放或其他预处理操作...
```
3. **检测车辆**:
可能需要使用模板匹配、边缘检测或者更复杂的物体检测算法(如YOLOv3或SSD),这一步会返回车辆位置或计数:
```python
from template_matching import find_cars # 自定义的模板匹配函数
cars_in_frame = find_cars(gray_frame)
vehicle_count = len(cars_in_frame)
```
4. **计算速度**:
如果需要,还需要跟踪车辆并估计速度,可以利用连续帧之间的车辆位置变化。这里仅作演示,实际速度计算可能会更复杂:
```python
if previous_frame is not None and next_frame is not None:
car_positions = [car[0] for car in cars_in_frame]
# 假设车辆运动相对平滑,简单计算平均速度示例
speed_estimate = calculate_speed(car_positions, previous_car_positions)
else:
speed_estimate = None
previous_car_positions = car_positions
```
5. **存储数据**:
每分钟收集的数据可以存入字典或列表中,然后定期(例如每60秒)将其写入CSV文件:
```python
time_data = {
'time': current_timestamp,
'vehicle_count': vehicle_count,
'speed_estimate': speed_estimate,
}
if len(time_data['time']) % 60 == 0: # 每60秒记录一次
with open('data.csv', 'a') as f:
writer = csv.DictWriter(f, fieldnames=time_data.keys())
writer.writerow(time_data)
```
6. **结束循环并释放资源**:
当遍历完视频文件后,关闭摄像头和保存文件:
```python
cap.release()
cv2.destroyAllWindows()
```
注意,上述代码简化了实际应用中的许多细节,如错误处理、性能优化和更精确的速度计算。实际项目中可能需要根据具体需求进行调整。
相关推荐
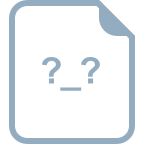
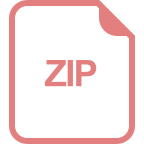
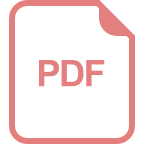














