lstm设计思路python
时间: 2023-11-07 19:06:16 浏览: 137
LSTM是一种循环神经网络,它可以处理和预测时间序列数据。在Python中,我们可以使用TensorFlow或Keras等库来实现LSTM模型。下面是LSTM模型的设计思路:
1. 导入所需的库和数据集。
```python
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, LSTM
from sklearn.model_selection import train_test_split
import numpy as np
# 导入数据集
data = np.array([[0.1, 0.2, 0.3], [0.2, 0.3, 0.4], [0.3, 0.4, 0.5], [0.4, 0.5, 0.6], [0.5, 0.6, 0.7]])
target = np.array([0.4, 0.5, 0.6, 0.7, 0.8])
```
2. 将数据集分为训练集和测试集。
```python
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(data, target, test_size=0.2, random_state=0)
```
3. 定义LSTM模型。
```python
# 定义LSTM模型
model = Sequential()
model.add(LSTM(50, activation='relu', input_shape=(3, 1)))
model.add(Dense(1))
```
4. 编译模型并训练。
```python
# 编译模型并训练
model.compile(optimizer='adam', loss='mse')
model.fit(X_train.reshape((X_train.shape[0], X_train.shape[1], 1)), y_train, epochs=200, verbose=0)
```
5. 评估模型并进行预测。
```python
# 评估模型并进行预测
mse = model.evaluate(X_test.reshape((X_test.shape[0], X_test.shape[1], 1)), y_test, verbose=0)
print('Mean Squared Error:', mse)
prediction = model.predict(np.array([[0.6, 0.7, 0.8]]).reshape((1, 3, 1)))
print('Prediction:', prediction[0][0])
```
阅读全文
相关推荐
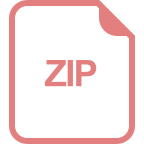
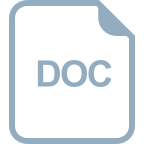

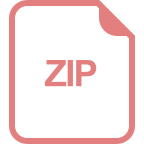
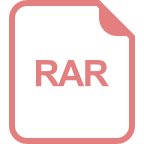
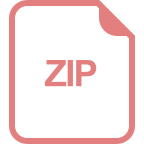
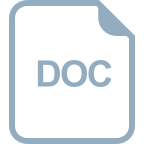
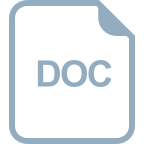
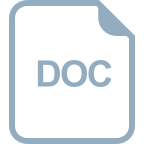
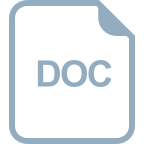
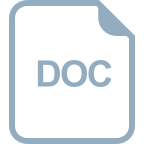
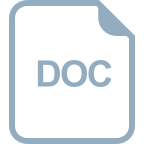
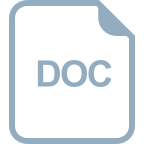
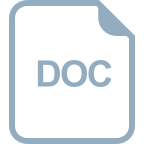
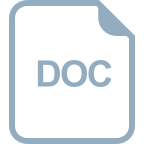
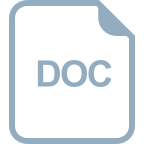