Python编程实现线性表两种存储结构中的基本操作的实现(线性表的创建、插入、删除和查找),并设计一个主菜单完成各个功能的调用。
时间: 2024-11-06 22:35:33 浏览: 23
在Python中,我们可以使用两种常见的数据结构来实现线性表:列表(list)和数组(array),这里我们分别讲解这两种结构的基本操作:
**1. 列表(List)实现:**
```python
class LinkedList:
def __init__(self):
self.head = None
# 创建列表
def create_list(self):
print("请输入元素,输入'q'结束:")
self.head = Node(int(input()))
# 插入元素
def insert(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
# 删除元素
def delete(self, key):
if self.head is None:
return
if self.head.data == key:
self.head = self.head.next
return
current = self.head
while current.next and current.next.data != key:
current = current.next
if current.next:
current.next = current.next.next
# 查找元素
def search(self, key):
current = self.head
while current:
if current.data == key:
return True
current = current.next
return False
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
# 主菜单
def main_menu():
print("1. 创建线性表")
print("2. 插入元素")
print("3. 删除元素")
print("4. 查找元素")
print("5. 退出")
choice = int(input("请输入您的选择: "))
if choice == 1:
linked_list = LinkedList()
linked_list.create_list()
elif choice == 2:
linked_list = LinkedList() if hasattr(linked_list, 'head') else LinkedList()
data = int(input("请输入插入的数据: "))
linked_list.insert(data)
elif choice == 3:
linked_list = LinkedList() if hasattr(linked_list, 'head') else LinkedList()
key = int(input("请输入要删除的数据: "))
linked_list.delete(key)
elif choice == 4:
linked_list = LinkedList() if hasattr(linked_list, 'head') else LinkedList()
key = int(input("请输入要查找的数据: "))
result = linked_list.search(key)
if result:
print(f"找到,数据 {key} 存在于链表中.")
else:
print(f"未找到数据 {key}.")
elif choice == 5:
exit(0)
if __name__ == "__main__":
main_menu()
```
**2. 数组(Array)实现:**
由于Python的标准库中没有原生支持动态数组的功能,我们需要第三方库如`numpy`来实现类似数组的操作。这里假设我们有一个名为`Array`的类。
```python
import numpy as np
class Array:
def __init__(self, size=10):
self.data = np.zeros(size, dtype=int)
# 创建数组
def create_array(self):
self.size = int(input("请输入数组大小: "))
self.data = np.zeros(self.size, dtype=int)
# 插入元素
def insert(self, index, data):
if index < 0 or index >= self.size:
print("插入位置无效.")
else:
self.data[index] = data
# 删除元素
def delete(self, index):
if index < 0 or index >= self.size:
print("删除位置无效.")
else:
self.data[index] = np.nan # 使用NaN填充删除的位置
# 查找元素
def search(self, key):
return np.isnan(self.data).sum() == 0 and np.any(self.data == key)
# ... (主菜单部分保持不变)
```
请注意,以上数组实现并不完美,因为`np.nan`不是有效的整数值,所以用于删除元素。实际应用中,可以考虑使用其他方法替代。
阅读全文
相关推荐
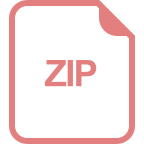
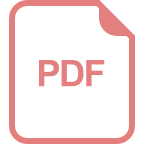
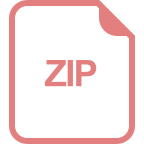

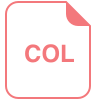
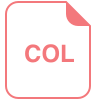
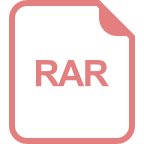
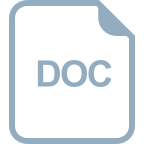
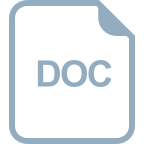
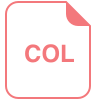
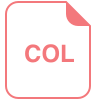
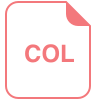
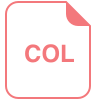
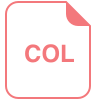
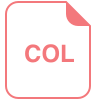
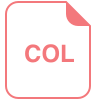
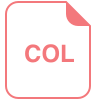
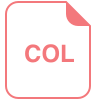
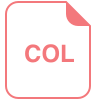