要求C语言编写:将一个带头结点的数据域依次为al,a2,…,an(n≥3)的单链表的所有结点逆置,即第一个结点的数据域变为an,…,最后一个结点的数据域为 al。编程实现以上功能要求。
时间: 2024-06-19 10:04:26 浏览: 13
要求编写C语言程序实现将一个带头结点的单链表逆置,即将链表的所有结点的数据域依次为al,a2,…,an(n≥3)的单链表的所有结点逆置,即第一个结点的数据域变为an,…,最后一个结点的数据域为 al。具体实现可以参考以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
// 创建链表
Node* createList(int n) {
Node *head, *p, *pre;
head = (Node*)malloc(sizeof(Node));
head->next = NULL;
pre = head;
int i;
for (i = 1; i <= n; i++) {
p = (Node*)malloc(sizeof(Node));
printf("请输入第%d个节点的值:", i);
scanf("%d", &p->data);
p->next = NULL;
pre->next = p;
pre = p;
}
return head;
}
// 遍历链表
void traverseList(Node *head) {
Node *p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 逆置链表
void reverseList(Node *head) {
Node *pre = NULL, *cur = head->next, *temp;
while (cur != NULL) {
temp = cur->next;
cur->next = pre;
pre = cur;
cur = temp;
}
head->next = pre;
}
int main() {
int n;
printf("请输入链表的长度:");
scanf("%d", &n);
Node *head = createList(n);
printf("原始链表:");
traverseList(head);
reverseList(head);
printf("逆置后的链表:");
traverseList(head);
return 0;
}
```
以上代码中,首先通过`createList()`函数创建带头结点的单链表,并通过`traverseList()`函数遍历链表。然后通过`reverseList()`函数实现逆置链表的功能,并再次调用`traverseList()`函数输出逆置后的链表。
相关推荐
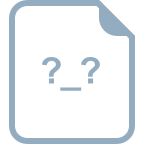













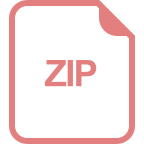