opencv轮廓最大外接矩形
时间: 2024-10-06 07:02:33 浏览: 59
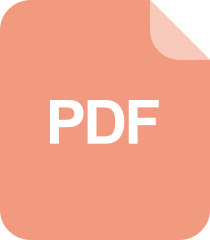
Opencv绘制最小外接矩形、最小外接圆
OpenCV(开源计算机视觉库)提供了一个功能,可以用来找到图像中轮廓的最大外接矩形。当你对图像中的边缘、物体或其他特征检测出轮廓后,轮廓最大外接矩形可以帮助你确定该轮廓最接近的正方形或长方形形状。这个矩形通常是通过计算轮廓边界点的最小和最大X坐标以及Y坐标来确定的。
在Python的OpenCV中,你可以使用`cv2.boundingRect()`函数来获取轮廓的矩形框信息。例如:
```python
import cv2
# 加载图像并进行边缘检测
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
_, contours, _ = cv2.findContours(img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
x, y, w, h = cv2.boundingRect(contour) # (x, y) - 矩形左上角坐标,w - 宽度,h - 高度
rect_max = (x, y, max(w, h), min(w, h)) # 最大外接矩形,保证宽高比接近1
print(rect_max)
```
阅读全文
相关推荐
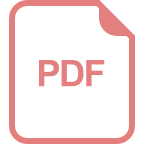
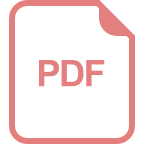
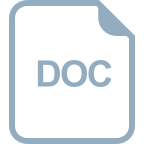
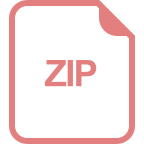
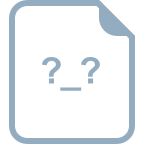
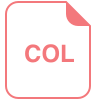
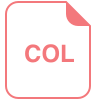










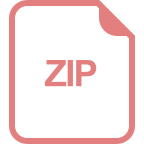