如何利用django连接mysql数据库并使用sql语句进行数据提取
时间: 2024-05-06 20:17:18 浏览: 8
要连接 MySQL 数据库并使用 SQL 语句进行数据提取,你需要在 Django 中进行以下配置:
1. 安装 MySQL 驱动程序
```
pip install mysql-connector-python
```
2. 在 `settings.py` 文件中配置数据库
```python
DATABASES = {
'default': {
'ENGINE': 'mysql.connector.django',
'NAME': 'your_database_name',
'USER': 'your_username',
'PASSWORD': 'your_password',
'HOST': 'your_host',
'PORT': 'your_port',
}
}
```
其中,`your_database_name` 是你要连接的数据库名称,`your_username` 是数据库用户名,`your_password` 是数据库密码,`your_host` 是数据库主机地址,`your_port` 是数据库端口号。
3. 在应用程序的 `views.py` 文件中编写 SQL 查询语句,例如:
```python
from django.db import connection
def your_view(request):
with connection.cursor() as cursor:
cursor.execute("SELECT * FROM your_table_name;")
rows = cursor.fetchall()
return render(request, 'your_template.html', {'rows': rows})
```
其中,`your_table_name` 是你要查询的表名称,`rows` 是查询结果。
4. 在 `your_template.html` 中显示查询结果,例如:
```html
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
{% for row in rows %}
<tr>
<td>{{ row.0 }}</td>
<td>{{ row.1 }}</td>
<td>{{ row.2 }}</td>
</tr>
{% endfor %}
</tbody>
</table>
```
其中,`row.0`、`row.1` 和 `row.2` 分别是查询结果中的第一列、第二列和第三列。
这样,你就可以使用 Django 连接 MySQL 数据库并使用 SQL 语句进行数据提取了。
相关推荐
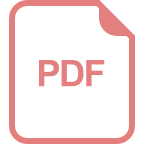
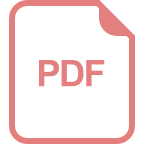
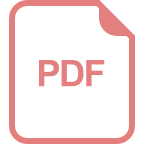
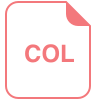
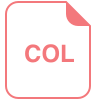
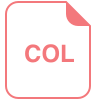
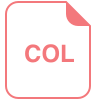










