怎么双击对treeview进行编辑
时间: 2023-12-17 17:03:57 浏览: 57
要实现双击编辑 Treeview 中的项目,你需要使用 Tkinter Treeview 组件的 `bind` 方法来绑定双击事件,并在事件处理函数中启用编辑功能。
以下是一个示例代码,可以实现双击 Treeview 中的项目进行编辑:
```python
import tkinter as tk
from tkinter import ttk
class EditableTreeview(ttk.Treeview):
def __init__(self, parent, **kwargs):
super().__init__(parent, **kwargs)
self.bind('<Double-1>', self.start_editing)
def start_editing(self, event):
"""Start editing the item on double click"""
# Get the item that was clicked
item_id = self.identify_row(event.y)
# Make sure an item was clicked and not the Treeview itself
if item_id:
# Get the text of the item that was clicked
item_text = self.item(item_id)['text']
# Create an Entry widget to edit the item
entry = tk.Entry(self, validate='key')
entry.insert(0, item_text)
# Set the Entry widget as the editor of the Treeview item
self.item(item_id, text='', image='',
tags=('editing',))
self.set(item_id, column=0, value='',
text='', image='',
tags=('editing',))
self.editing_id = item_id
self.editing_widget = entry
self.editing_value = item_text
# Place the Entry widget in the Treeview
self.pack_forget()
self.place(in_=self, relx=1, anchor='w',
x=self.bbox(item_id, column=0)['x'],
y=self.bbox(item_id)['y'])
entry.focus_set()
entry.bind('<Return>', self.finish_editing)
entry.bind('<Escape>', self.cancel_editing)
entry.bind('<FocusOut>', self.cancel_editing)
def finish_editing(self, event):
"""Finish editing the item"""
new_value = self.editing_widget.get()
self.set(self.editing_id, column=0, value=new_value)
self.item(self.editing_id, text=new_value, image='',
tags=('edited',))
self.editing_widget.destroy()
self.editing_id = None
self.editing_widget = None
self.editing_value = None
def cancel_editing(self, event):
"""Cancel editing the item"""
self.set(self.editing_id, column=0, value=self.editing_value)
self.item(self.editing_id, text=self.editing_value, image='',
tags=('cancelled',))
self.editing_widget.destroy()
self.editing_id = None
self.editing_widget = None
self.editing_value = None
# Create the Treeview widget
root = tk.Tk()
treeview = EditableTreeview(root)
treeview.pack()
# Insert some items into the Treeview
treeview.insert('', 'end', text='Item 1')
treeview.insert('', 'end', text='Item 2')
treeview.insert('', 'end', text='Item 3')
# Start the main event loop
root.mainloop()
```
在这个示例中,我们创建了一个名为 `EditableTreeview` 的自定义 Treeview 组件,它继承自 Tkinter Treeview 组件,并覆盖了其 `__init__` 方法和 `start_editing` 方法。在 `__init__` 方法中,我们使用 `bind` 方法来绑定 `<Double-1>` 事件,即双击事件,当用户双击 Treeview 中的项目时,`start_editing` 方法会被调用。
在 `start_editing` 方法中,我们首先获取被点击的项目的 ID,然后创建一个 `Entry` 组件来编辑该项目。我们将 `Entry` 组件作为 Treeview 项目的编辑器,并将其放置在 Treeview 中。在 `Entry` 组件中,我们使用 `<Return>`、`<Escape>` 和 `<FocusOut>` 事件来完成、取消或离开编辑模式。
在 `finish_editing` 方法中,我们获取编辑器中的新值,并将其设置为 Treeview 项目的新值。在 `cancel_editing` 方法中,我们将编辑器中的值恢复为原始值。
最后,我们创建了一个 `EditableTreeview` 实例,并在其中插入了一些项目。运行程序后,你应该可以双击 Treeview 中的项目,并使用 `Entry` 组件编辑该项目。
相关推荐
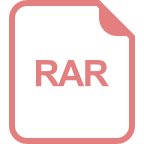
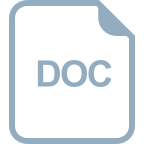














