帮我用Java写一个吃豆人小游戏
时间: 2024-01-25 08:04:56 浏览: 126
好的,我可以给您提供一个简单的吃豆人小游戏的Java实现,但需要您明确一下您对游戏的要求和期望,例如游戏界面、游戏规则、游戏操作等。以下是一个简单的示例代码,您可以参考一下:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class PacMan extends JPanel implements KeyListener {
private static final long serialVersionUID = 1L;
private static final int BLOCK_SIZE = 20;
private static final int MARGIN_SIZE = 20;
private static final int ROWS = 20;
private static final int COLS = 20;
private static final int PACMAN_SPEED = 3;
private static final int GHOST_SPEED = 2;
private static final int DELAY = 20;
private static final int SCORE_DOT = 10;
private static final int SCORE_GHOST = 50;
private static final int SCORE_CHERRY = 100;
private static final int CHERRY_INTERVAL = 20;
private enum Direction {
LEFT, UP, RIGHT, DOWN
}
private Point pacman;
private Direction pacmanDirection;
private List<Point> ghosts;
private List<Direction> ghostDirections;
private List<Point> dots;
private int score;
private int cherryCountdown;
private boolean gameOver;
private boolean gameWon;
public PacMan() {
pacman = new Point(ROWS / 2, COLS / 2);
pacmanDirection = Direction.LEFT;
ghosts = new ArrayList<>();
ghostDirections = new ArrayList<>();
for (int i = 0; i < 4; i++) {
ghosts.add(new Point(ROWS / 2 + i, COLS / 2));
ghostDirections.add(Direction.LEFT);
}
dots = new ArrayList<>();
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if ((i == 0 || i == ROWS - 1 || j == 0 || j == COLS - 1) && (i != ROWS / 2 || j < COLS / 2 - 2 || j > COLS / 2 + 1)) {
dots.add(new Point(i, j));
}
}
}
score = 0;
cherryCountdown = CHERRY_INTERVAL;
gameOver = false;
gameWon = false;
}
public void run() {
JFrame frame = new JFrame("Pac-Man");
frame.setSize(ROWS * BLOCK_SIZE + 2 * MARGIN_SIZE, COLS * BLOCK_SIZE + 2 * MARGIN_SIZE);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLocationRelativeTo(null);
frame.addKeyListener(this);
frame.getContentPane().add(this);
frame.setVisible(true);
while (!gameOver && !gameWon) {
update();
repaint();
try {
Thread.sleep(DELAY);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
if (gameOver) {
System.out.println("Game over!");
} else if (gameWon) {
System.out.println("Congratulations, you won!");
}
}
private void update() {
movePacman();
moveGhosts();
checkCollisions();
checkWinningCondition();
updateCherryCountdown();
}
private void movePacman() {
Point next = getNextPoint(pacman, pacmanDirection);
if (dots.contains(next)) {
dots.remove(next);
score += SCORE_DOT;
}
if (cherryCountdown == 0 && next.equals(getRandomPoint())) {
score += SCORE_CHERRY;
cherryCountdown = CHERRY_INTERVAL;
}
if (!isWall(next)) {
pacman = next;
}
}
private void moveGhosts() {
for (int i = 0; i < ghosts.size(); i++) {
Point ghost = ghosts.get(i);
Direction direction = ghostDirections.get(i);
Point next = getNextPoint(ghost, direction);
if (next.equals(pacman)) {
gameOver = true;
return;
}
if (isWall(next) || ghosts.contains(next)) {
direction = getRandomDirection();
}
ghosts.set(i, getNextPoint(ghost, direction));
ghostDirections.set(i, direction);
}
}
private void checkCollisions() {
for (Point ghost : ghosts) {
if (ghost.equals(pacman)) {
gameOver = true;
return;
}
}
}
private void checkWinningCondition() {
if (dots.isEmpty()) {
gameWon = true;
}
}
private void updateCherryCountdown() {
cherryCountdown = Math.max(0, cherryCountdown - 1);
}
private Point getNextPoint(Point point, Direction direction) {
switch (direction) {
case LEFT:
return new Point(point.x, point.y - 1);
case UP:
return new Point(point.x - 1, point.y);
case RIGHT:
return new Point(point.x, point.y + 1);
case DOWN:
return new Point(point.x + 1, point.y);
default:
return point;
}
}
private boolean isWall(Point point) {
return point.x < 0 || point.x >= ROWS || point.y < 0 || point.y >= COLS ||
(point.x == ROWS / 2 && point.y >= COLS / 2 - 2 && point.y <= COLS / 2 + 1) ||
(point.x == 0 && point.y == COLS / 2) || (point.x == ROWS - 1 && point.y == COLS / 2);
}
private Point getRandomPoint() {
while (true) {
Point point = new Point(new Random().nextInt(ROWS - 2) + 1, new Random().nextInt(COLS - 2) + 1);
if (!isWall(point) && !dots.contains(point) && !ghosts.contains(point) && !point.equals(pacman)) {
return point;
}
}
}
private Direction getRandomDirection() {
Direction[] directions = Direction.values();
return directions[new Random().nextInt(directions.length)];
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.BLACK);
g.fillRect(0, 0, getWidth(), getHeight());
g.setColor(Color.WHITE);
g.drawString("Score: " + score, MARGIN_SIZE, MARGIN_SIZE - 5);
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (dots.contains(new Point(i, j))) {
g.setColor(Color.YELLOW);
g.fillOval(MARGIN_SIZE + j * BLOCK_SIZE + BLOCK_SIZE / 2 - 2, MARGIN_SIZE + i * BLOCK_SIZE + BLOCK_SIZE / 2 - 2, 4, 4);
} else if (i == ROWS / 2 && j >= COLS / 2 - 2 && j <= COLS / 2 + 1) {
g.setColor(Color.BLUE);
g.fillRect(MARGIN_SIZE + j * BLOCK_SIZE, MARGIN_SIZE + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
} else if (ghosts.contains(new Point(i, j))) {
g.setColor(Color.RED);
g.fillOval(MARGIN_SIZE + j * BLOCK_SIZE + BLOCK_SIZE / 2 - 8, MARGIN_SIZE + i * BLOCK_SIZE + BLOCK_SIZE / 2 - 8, 16, 16);
} else if (pacman.equals(new Point(i, j))) {
g.setColor(Color.YELLOW);
g.fillArc(MARGIN_SIZE + j * BLOCK_SIZE, MARGIN_SIZE + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE, pacmanDirection.ordinal() * 90 + 30, 300);
}
}
}
if (cherryCountdown == 0) {
g.setColor(Color.RED);
Point cherry = getRandomPoint();
g.fillOval(MARGIN_SIZE + cherry.y * BLOCK_SIZE + BLOCK_SIZE / 2 - 5, MARGIN_SIZE + cherry.x * BLOCK_SIZE + BLOCK_SIZE / 2 - 5, 10, 10);
}
}
@Override
public void keyPressed(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_LEFT:
pacmanDirection = Direction.LEFT;
break;
case KeyEvent.VK_UP:
pacmanDirection = Direction.UP;
break;
case KeyEvent.VK_RIGHT:
pacmanDirection = Direction.RIGHT;
break;
case KeyEvent.VK_DOWN:
pacmanDirection = Direction.DOWN;
break;
}
}
@Override
public void keyReleased(KeyEvent e) {
}
@Override
public void keyTyped(KeyEvent e) {
}
public static void main(String[] args) {
new PacMan().run();
}
}
```
该实现使用Java Swing绘制游戏界面,并提供了简单的游戏规则和操作。您可以根据自己的需求对代码进行修改和扩展。希望对您有所帮助!
阅读全文
相关推荐
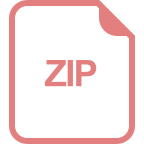
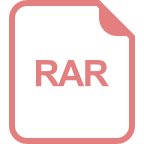
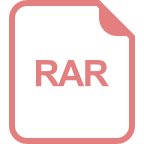
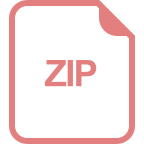
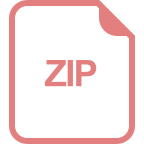
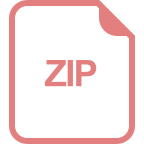
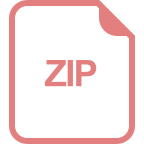
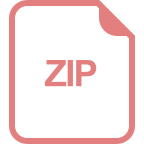
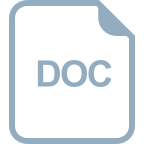
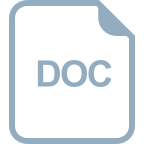
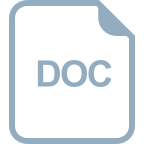
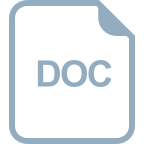
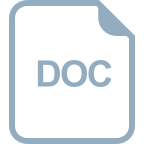
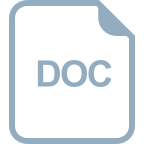
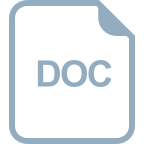