用Python写一个管理系统
时间: 2024-03-30 18:15:42 浏览: 111
好的,管理系统的功能有很多种,可以是商品管理、订单管理、员工管理等等。这里我以商品管理系统为例,给出一个简单的Python代码。
首先是需要用到的库和数据:
```python
import csv
# 商品数据
products = [
{"id": 1, "name": "Apple", "price": 5},
{"id": 2, "name": "Banana", "price": 10},
{"id": 3, "name": "Orange", "price": 7},
]
# 数据存储文件
filename = "products.csv"
```
接下来是需要实现的功能:
1. 显示全部商品信息
2. 添加新商品
3. 修改商品信息
4. 删除商品
```python
def show_products():
"""显示所有商品信息"""
print("ID\tName\tPrice")
for product in products:
print(f"{product['id']}\t{product['name']}\t{product['price']}")
print()
def add_product():
"""添加新商品"""
name = input("请输入商品名称:")
price = float(input("请输入商品价格:"))
# 获取最大ID
max_id = max(products, key=lambda x: x["id"])["id"]
product = {"id": max_id + 1, "name": name, "price": price}
products.append(product)
save_data()
def modify_product():
"""修改商品信息"""
id = int(input("请输入要修改的商品ID:"))
product = next((p for p in products if p["id"] == id), None)
if product:
print(f"当前商品信息为:{product['name']} {product['price']}")
name = input("请输入新的商品名称:")
price = float(input("请输入新的商品价格:"))
product["name"] = name
product["price"] = price
save_data()
else:
print(f"未找到ID为{id}的商品!")
def delete_product():
"""删除商品"""
id = int(input("请输入要删除的商品ID:"))
product = next((p for p in products if p["id"] == id), None)
if product:
products.remove(product)
save_data()
print(f"已删除ID为{id}的商品!")
else:
print(f"未找到ID为{id}的商品!")
def save_data():
"""保存数据到文件"""
with open(filename, "w", newline="") as csvfile:
fieldnames = ["id", "name", "price"]
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for product in products:
writer.writerow(product)
def load_data():
"""从文件加载数据"""
try:
with open(filename, newline="") as csvfile:
reader = csv.DictReader(csvfile)
products.clear()
for row in reader:
product = {"id": int(row["id"]), "name": row["name"], "price": float(row["price"])}
products.append(product)
except FileNotFoundError:
pass
# 加载数据
load_data()
# 主循环
while True:
print("1. 显示所有商品信息")
print("2. 添加新商品")
print("3. 修改商品信息")
print("4. 删除商品")
print("5. 退出系统")
choice = input("请输入操作编号:")
if choice == "1":
show_products()
elif choice == "2":
add_product()
elif choice == "3":
modify_product()
elif choice == "4":
delete_product()
elif choice == "5":
break
else:
print("输入有误,请重新输入!")
```
这个管理系统使用了CSV文件来存储数据,并提供了显示、添加、修改、删除等功能。你可以根据实际需求进行扩展或修改。
阅读全文
相关推荐
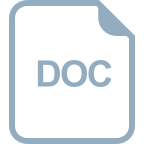
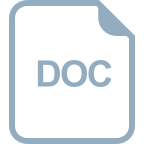
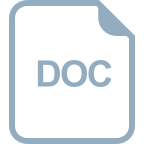
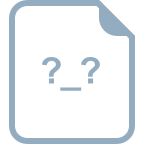
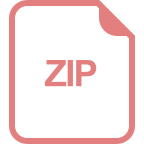
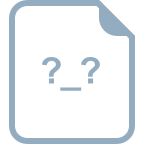
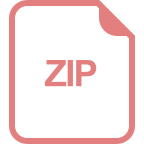
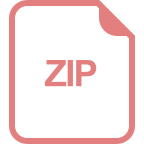
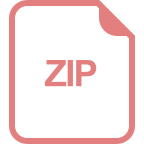
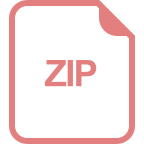
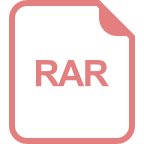
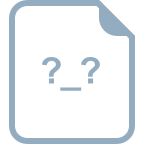
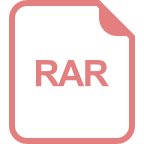
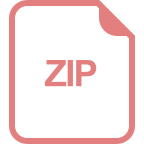
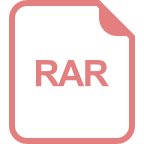
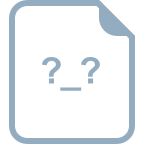
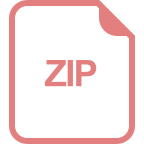