c#窗体应用程序实现软件开机自启,并可以通过路径控制ICT测试软件的打开和关闭。良品条码和不良品条码可以在本地文件夹中的txt文件中获取。当软件开启,定时器就在数据库扫描是否有设置的良品和不良品条码的当天测试记录,如果存在,lable状态栏变绿并提示“以点检,请进行测试”就可以通过c#软件开启按钮打开ICT测试软件,进行别的条码的ICT测试。如果没有,点击开启ICT测试按钮时,label状态栏变红,并提示“请进行测试前点检”,然后就先进行设置条码的ICT测试,判断扫描到的条码是否为组件中设置的良品或不良品条码,如输入的条码不符合,label状态栏变红,并提示“条码错误”,如输入的条码正确并为设置的良品条码,label状态栏变绿,并提升“请进行良品点检”,这时就将扫到的良品条码通过模拟键盘复制黏贴到ICT条码栏,进行ICT测试,测试完成后就提示“良品点检完成”
时间: 2023-11-09 22:07:38 浏览: 231
实现软件开机自启可以通过在应用程序的 Program.cs 文件中添加以下代码:
```csharp
static void Main()
{
if (!System.Diagnostics.EventLog.SourceExists("MyApp"))
{
System.Diagnostics.EventLog.CreateEventSource("MyApp", "Application");
}
// 判断是否第一次打开应用程序
if (Properties.Settings.Default.FirstRun)
{
// 设置开机自启
Microsoft.Win32.RegistryKey rk = Microsoft.Win32.Registry.CurrentUser.OpenSubKey("SOFTWARE\\Microsoft\\Windows\\CurrentVersion\\Run", true);
rk.SetValue("MyApp", Application.ExecutablePath.ToString());
// 将 FirstRun 设为 false
Properties.Settings.Default.FirstRun = false;
Properties.Settings.Default.Save();
}
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
}
```
控制ICT测试软件的打开和关闭可以通过使用 Process 类来实现。以下是一个示例代码:
```csharp
// 打开软件
Process.Start("C:\\path\\to\\software.exe");
// 关闭软件
foreach (Process process in Process.GetProcessesByName("software"))
{
process.Kill();
}
```
定时器扫描数据库可以使用 System.Timers.Timer 类来实现。以下是一个示例代码:
```csharp
private System.Timers.Timer timer;
public MainForm()
{
InitializeComponent();
// 初始化定时器
timer = new System.Timers.Timer();
timer.Interval = 60000; // 1 分钟扫描一次
timer.Elapsed += new ElapsedEventHandler(OnTimedEvent);
timer.AutoReset = true;
timer.Enabled = true;
}
private void OnTimedEvent(object source, ElapsedEventArgs e)
{
// 扫描数据库
bool hasRecord = CheckDatabase();
// 更新 label 状态栏
if (hasRecord)
{
labelStatus.Text = "以点检,请进行测试";
labelStatus.ForeColor = Color.Green;
}
else
{
labelStatus.Text = "请进行测试前点检";
labelStatus.ForeColor = Color.Red;
}
}
```
判断扫描到的条码是否为组件中设置的良品或不良品条码可以将良品和不良品条码保存到数组中,然后使用 Array.Contains() 方法进行判断。以下是一个示例代码:
```csharp
string[] goodBarcodes = File.ReadAllLines("C:\\path\\to\\good_barcodes.txt");
string[] badBarcodes = File.ReadAllLines("C:\\path\\to\\bad_barcodes.txt");
private void ButtonTest_Click(object sender, EventArgs e)
{
string barcode = textBoxBarcode.Text.Trim();
if (goodBarcodes.Contains(barcode))
{
labelStatus.Text = "请进行良品点检";
labelStatus.ForeColor = Color.Green;
// 将扫到的良品条码通过模拟键盘复制黏贴到ICT条码栏
SendKeys.Send("^a");
SendKeys.Send("^c");
SendKeys.SendWait("{TAB}");
SendKeys.SendWait("^v");
SendKeys.SendWait("{ENTER}");
MessageBox.Show("良品点检完成");
}
else if (badBarcodes.Contains(barcode))
{
labelStatus.Text = "请进行不良品点检";
labelStatus.ForeColor = Color.Yellow;
// 进行不良品测试
}
else
{
labelStatus.Text = "条码错误";
labelStatus.ForeColor = Color.Red;
}
}
```
阅读全文
相关推荐
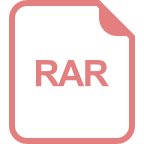
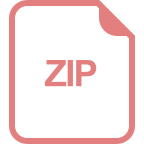
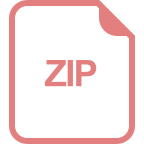




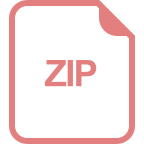