datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S')返回值为字符串,如何将其转换成%f的数据类型
时间: 2024-06-08 15:04:18 浏览: 20
`datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S')` 是用来将当前日期和时间格式化为 '年-月-日 时:分:秒' 格式的字符串。`%f` 是一个精度为微秒的格式代码,但在 Python 的 `strftime` 方法中,它并不是直接支持的。如果你想获取微秒级别的精确时间,你需要在其他地方进行转换。
Python datetime 对象本身并不直接提供 `%f` 格式,但你可以先将字符串转换为 `datetime` 对象,然后使用 `.microsecond` 属性获取微秒值。以下是一个示例:
```python
from datetime import datetime
# 获取当前时间的字符串
str_time = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
# 将字符串转换回datetime对象
dt_obj = datetime.strptime(str_time, '%Y-%m-%d %H:%M:%S')
# 提取微秒部分
microseconds = dt_obj.microsecond
# 如果你想转换为浮点数表示,微秒乘以10**6(因为 Python 的微秒是整数)
float_microseconds = microseconds / 10**6
print(float_microseconds)
```
相关问题
优化以下ptyhon代码import datetime import time import matplotlib.pyplot as plt # 初始化图形 plt.ion() # 时间列表和高度列表 time_list = [] height_list = [] # 创建柱状图 fig, ax = plt.subplots() # 设置图形初始属性 ax.set_xlabel('Time') ax.set_ylabel('Height') ax.set_title('Real-time Bar Chart') # 开始循环 while True: # 获取当前时间和高度 current_time = datetime.datetime.now().strftime("%H:%M:%S") current_height = datetime.datetime.now().second # 添加时间和高度到列表 time_list.append(current_time) height_list.append(current_height) # 清空图形 ax.clear() # 绘制柱状图 ax.bar(time_list, height_list) # 设置x轴标签旋转角度 plt.xticks(rotation=45) # 刷新图形 plt.draw() plt.pause(0.1)
这段 Python 代码的功能是实时绘制柱状图,显示当前时间和高度信息。
以下是对该代码进行优化的建议:
1. 减少重复获取时间的次数:在当前代码中,每次循环都会调用 `datetime.datetime.now()` 来获取当前时间。可以在循环开始时获取一次当前时间,并在后续的循环中直接使用该变量。
2. 使用动态更新的方式绘制柱状图:当前代码每次循环都会清空图形并重新绘制柱状图,这样会导致频繁的图形重绘和刷新。可以使用 `ax.bar` 方法的返回值来获取柱状图对象,并在后续的循环中更新该对象的数据,而不是重新绘制整个图形。
3. 优化图形刷新频率:当前代码中使用 `plt.pause(0.1)` 来暂停程序执行,以实现动态更新图形。可以考虑根据实际需求调整刷新频率,避免过快或过慢的刷新。
下面是优化后的代码示例:
```python
import datetime
import time
import matplotlib.pyplot as plt
# 初始化图形
plt.ion()
# 时间列表和高度列表
time_list = []
height_list = []
# 创建柱状图
fig, ax = plt.subplots()
# 设置图形初始属性
ax.set_xlabel('Time')
ax.set_ylabel('Height')
ax.set_title('Real-time Bar Chart')
# 获取初始时间
current_time = datetime.datetime.now().strftime("%H:%M:%S")
# 开始循环
while True:
# 获取当前高度
current_height = datetime.datetime.now().second
# 添加时间和高度到列表
time_list.append(current_time)
height_list.append(current_height)
# 更新柱状图数据
ax.bar(time_list, height_list)
# 设置x轴标签旋转角度
plt.xticks(rotation=45)
# 刷新图形
plt.draw()
plt.pause(0.1)
# 更新当前时间
current_time = datetime.datetime.now().strftime("%H:%M:%S")
```
通过减少重复获取时间的次数、使用动态更新的方式绘制柱状图,并优化图形刷新频率,可以提高代码的效率和可读性。
def create_an_order(self,customer_id,product_id,create_time): is_created = False create_time = datetime.datetime.now().strftime("%Y-%m-%d_%H:%M:%S") with open('./data/user.txt','r') as f1: all_users = [eval(each.strip()) for each in f1.readlines()] all_ids = [item['user_id'] for item in all_users] with open('./data/produts.txt','r') as f2: all_pros = [eval(each.strip()) for each in f2.readlines()] all_pro_ids = [item['pro_id'] for item in all_pros] if customer_id in all_ids and product_id in all_pro_ids: order_id = self.generate_unique_order_id() orders = Order(order_id, customer_id, product_id,create_time) is_created = True with open('./data/orders.txt', 'a') as fw: fw.write(str(orders) + '\n') return is_created
这段代码实现了创建订单的功能,其中 `customer_id` 表示客户ID, `product_id` 表示商品ID, `create_time` 表示订单创建时间,函数返回值 `is_created` 表示订单是否创建成功。
具体实现步骤如下:
1. 将当前时间格式化为字符串形式。
```python
create_time = datetime.datetime.now().strftime("%Y-%m-%d_%H:%M:%S")
```
2. 读取用户信息和商品信息文件,将其转换为字典形式。
```python
with open('./data/user.txt', 'r') as f1:
all_users = [eval(each.strip()) for each in f1.readlines()]
with open('./data/produts.txt', 'r') as f2:
all_pros = [eval(each.strip()) for each in f2.readlines()]
```
3. 获取所有用户和商品的ID列表。
```python
all_ids = [item['user_id'] for item in all_users]
all_pro_ids = [item['pro_id'] for item in all_pros]
```
4. 判断 `customer_id` 和 `product_id` 是否存在于用户ID列表和商品ID列表中,如果存在则创建订单。
```python
if customer_id in all_ids and product_id in all_pro_ids:
order_id = self.generate_unique_order_id()
orders = Order(order_id, customer_id, product_id, create_time)
is_created = True
with open('./data/orders.txt', 'a') as fw:
fw.write(str(orders) + '\n')
```
5. 返回订单创建结果。
```python
return is_created
```
需要注意的是,这段代码中使用了 `eval()` 函数将文件中的字符串转换为字典,这种操作可能存在安全隐患,建议使用更加安全的方式进行文件读取和解析。另外, `generate_unique_order_id()` 函数没有给出具体实现,需要根据实际情况进行编写。
相关推荐
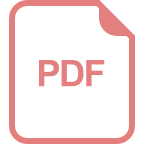
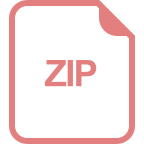









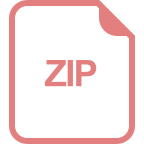
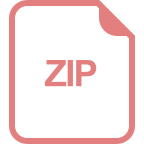