用C++写以上步骤
时间: 2023-08-15 14:03:07 浏览: 85
以下是用C++实现以上步骤的示例代码:
```cpp
#include <iostream>
#include <string>
#include <curl/curl.h>
#include <jsoncpp/json/json.h>
using namespace std;
// callback function for curl
size_t callback(char* ptr, size_t size, size_t nmemb, string* data) {
data->append(ptr, size * nmemb);
return size * nmemb;
}
int main() {
// set up curl
CURL* curl = curl_easy_init();
if (!curl) {
cerr << "Error: failed to initialize curl" << endl;
return 1;
}
// set up parameters
string url = "https://api.openai.com/v1/engines/davinci-codex/completions";
string prompt = "Hello,";
string api_key = "<YOUR_API_KEY_HERE>";
int max_tokens = 50;
double temperature = 0.5;
// encode parameters into json
Json::Value json_data;
json_data["prompt"] = prompt;
json_data["max_tokens"] = max_tokens;
json_data["temperature"] = temperature;
Json::Value json_headers;
json_headers["Content-Type"] = "application/json";
json_headers["Authorization"] = "Bearer " + api_key;
Json::Value json_request;
json_request["data"] = json_data;
json_request["headers"] = json_headers;
string json_string = json_request.toStyledString();
// set up curl options
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl, CURLOPT_POST, 1L);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, json_string.c_str());
curl_easy_setopt(curl, CURLOPT_POSTFIELDSIZE, json_string.length());
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, callback);
string response_body;
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &response_body);
// execute curl request
CURLcode res = curl_easy_perform(curl);
if (res != CURLE_OK) {
cerr << "Error: curl_easy_perform() failed: " << curl_easy_strerror(res) << endl;
curl_easy_cleanup(curl);
return 1;
}
// parse json response
Json::Value json_response;
Json::CharReaderBuilder builder;
Json::CharReader* reader = builder.newCharReader();
string errors;
if (!reader->parse(response_body.c_str(), response_body.c_str() + response_body.size(), &json_response, &errors)) {
cerr << "Error: failed to parse json response: " << errors << endl;
curl_easy_cleanup(curl);
return 1;
}
string completions = json_response["choices"][0]["text"].asString();
cout << completions << endl;
// clean up
delete reader;
curl_easy_cleanup(curl);
return 0;
}
```
这个程序使用了 `curl` 库来发送 HTTP 请求,使用了 `jsoncpp` 库来处理 JSON 数据。在运行这个程序之前需要先安装这两个库,并且将 `<YOUR_API_KEY_HERE>` 替换成你的 OpenAI API Key。
阅读全文
相关推荐
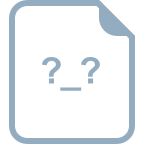
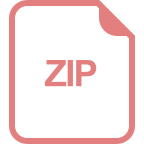
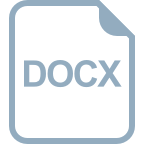


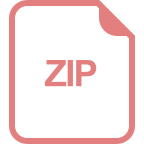
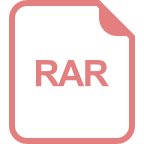
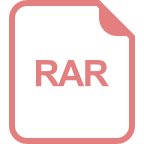
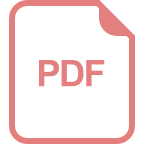
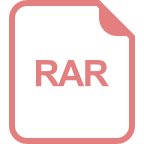
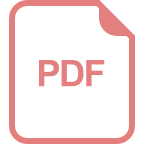
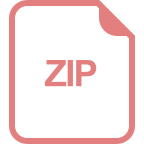
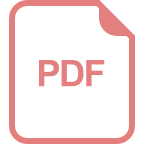
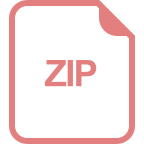