unity2D敌人在x轴和y轴移动追踪玩家不可斜角追踪自动躲避障碍物并且输出方向
时间: 2023-06-19 16:07:38 浏览: 33
你可以使用Unity的NavMeshAgent组件来实现敌人的移动和自动躲避障碍物。以下是实现步骤:
1. 添加NavMeshAgent组件到敌人对象上。
2. 设置NavMeshAgent的移动速度和角色的最大移动速度相匹配。
3. 在代码中,使用NavMeshAgent的SetDestination方法来设置敌人的目标位置,即玩家的位置。
4. 在Update方法中,使用NavMeshAgent的velocity属性获取敌人的速度向量,然后根据其x和y方向的值来确定敌人的移动方向。
5. 如果敌人检测到障碍物,则使用NavMeshAgent的AvoidancePriority属性来设置敌人的躲避优先级,以便它可以避免与障碍物碰撞。
6. 最后,你可以使用Debug.DrawRay方法来绘制从敌人位置开始的方向向量,以便你可以在场景中看到敌人的移动方向。
以下是一个基本示例代码:
```csharp
using UnityEngine;
using UnityEngine.AI;
public class EnemyController : MonoBehaviour
{
public Transform target;
public float moveSpeed = 5f;
public float rotationSpeed = 3f;
private NavMeshAgent agent;
private void Start()
{
agent = GetComponent<NavMeshAgent>();
agent.speed = moveSpeed;
agent.angularSpeed = rotationSpeed;
}
private void Update()
{
agent.SetDestination(target.position);
Vector3 direction = agent.velocity.normalized;
float xDir = direction.x;
float yDir = direction.z;
if (xDir != 0 || yDir != 0)
{
Debug.DrawRay(transform.position, direction, Color.red);
}
}
private void OnTriggerStay(Collider other)
{
if (other.CompareTag("Obstacle"))
{
agent.avoidancePriority = 50;
}
}
private void OnTriggerExit(Collider other)
{
if (other.CompareTag("Obstacle"))
{
agent.avoidancePriority = 0;
}
}
}
```
相关推荐
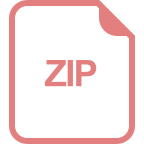


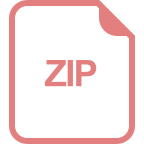
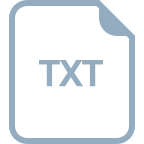
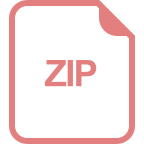
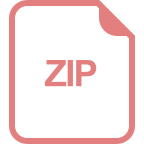
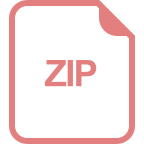
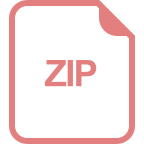
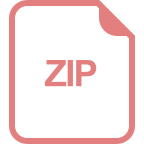
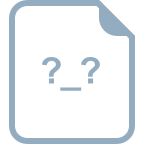