编写一个SpringBoot的Starter
时间: 2024-05-12 20:13:48 浏览: 78
Spring Boot Starter 是一种用于快速启动应用程序的方式,使得我们可以将一些常用的依赖项和配置组合到一起,以便于开发者可以更快速地启动项目。在本文中,我将介绍如何编写一个简单的 Spring Boot Starter。
首先,我们需要创建一个基本的 Maven 项目。在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-autoconfigure</artifactId>
<version>2.5.2</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>2.5.2</version>
</dependency>
```
这些依赖项包括 Spring Boot 的自动配置和启动器依赖项。
接下来,我们需要创建一个配置类,用于定义我们的 Starter 的属性。在 src/main/java 目录下创建一个类,例如 MyStarterProperties:
```java
@ConfigurationProperties(prefix = "mystarter")
public class MyStarterProperties {
private String message = "Hello, world!";
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
}
```
在上面的代码中,我们定义了一个名为 mystarter 的属性前缀,然后定义了一个名为 message 的属性,其默认值为 "Hello, world!"。
接下来,我们需要创建一个自动配置类,用于配置 Spring Boot 应用程序。在 src/main/java 目录下创建一个类,例如 MyStarterAutoConfiguration:
```java
@Configuration
@EnableConfigurationProperties(MyStarterProperties.class)
public class MyStarterAutoConfiguration {
private final MyStarterProperties properties;
public MyStarterAutoConfiguration(MyStarterProperties properties) {
this.properties = properties;
}
@Bean
@ConditionalOnMissingBean
public MyStarterService myStarterService() {
return new MyStarterService(properties.getMessage());
}
}
```
在上面的代码中,我们使用 @Configuration 注解将类标记为自动配置类,并使用 @EnableConfigurationProperties(MyStarterProperties.class) 注解将 MyStarterProperties 类添加到 Spring 环境中。然后,我们使用 @ConditionalOnMissingBean 注解检查是否已经存在名为 myStarterService 的 Bean。如果没有,则创建一个新的 MyStarterService Bean。
最后,我们需要编写一个服务类,它将使用我们的 Starter。在 src/main/java 目录下创建一个类,例如 MyStarterService:
```java
public class MyStarterService {
private final String message;
public MyStarterService(String message) {
this.message = message;
}
public void printMessage() {
System.out.println(message);
}
}
```
现在,我们已经完成了我们的 Starter。我们可以将其打包并发布到 Maven 中央仓库,以便其他开发者可以使用它。
对于其他开发者,他们只需要在他们的项目中添加以下依赖:
```xml
<dependency>
<groupId>com.example</groupId>
<artifactId>my-starter</artifactId>
<version>1.0.0</version>
</dependency>
```
然后,他们就可以像使用普通的 Spring Bean 一样使用我们的 Starter:
```java
@Autowired
private MyStarterService myStarterService;
// ...
myStarterService.printMessage();
```
这就是编写一个简单的 Spring Boot Starter 的过程。
阅读全文
相关推荐

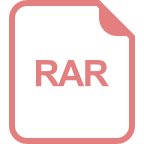
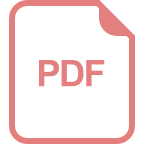
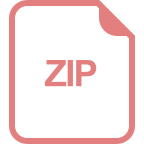
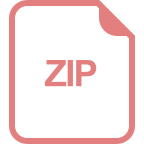
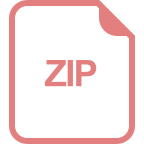
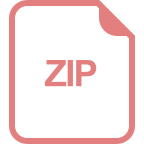
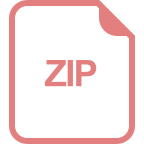
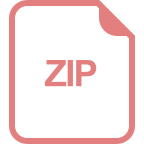
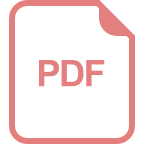
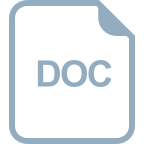
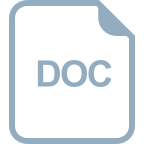
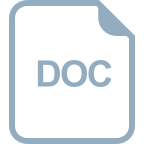
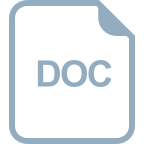

